Personal tools
num_get

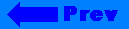
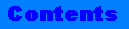
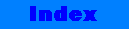
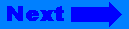
Click on the banner to return to the class reference home page.
num_get
num_getlocale::facet
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructor and Destructor
- Facet ID
- Public Member Functions
- Protected Member Functions
- Example
- See Also
Summary
Numeric formatting facet for input.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Synopsis
#include <locale> template <class charT, class InputIterator > class num_get;
Description
The num_get provides facilities for formatted input of numbers. basic_istream and all other input-oriented streams use this facet to implement formatted numeric input.
Interface
template <class charT, class InputIterator = istreambuf_iterator<charT> > class num_get : public locale::facet { public: typedef charT char_type; typedef InputIterator iter_type; explicit num_get(size_t refs = 0); iter_type get(iter_type, iter_type, ios_base&, ios_base::iostate&, bool&) const; iter_type get(iter_type, iter_type, ios_base& , ios_base::iostate&, long&) const; iter_type get(iter_type, iter_type, ios_base&, ios_base::iostate&, unsigned short&) const; iter_type get(iter_type, iter_type, ios_base&, ios_base::iostate&, unsigned int&) const; iter_type get(iter_type, iter_type, ios_base&, ios_base::iostate&, unsigned long&) const; iter_type get(iter_type, iter_type, ios_base&, ios_base::iostate&, float&) const; iter_type get(iter_type, iter_type, ios_base&, ios_base::iostate&, double&) const; iter_type get(iter_type, iter_type, ios_base&, ios_base::iostate&, long double&) const; static locale::id id; protected: ~num_get(); // virtual virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, bool&) const; virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, long&) const; virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, unsigned short&) const; virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, unsigned int&) const; virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, unsigned long&) const; virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, float&) const; virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, double&) const; virtual iter_type do_get(iter_type, iter_type, ios_base&, ios_base::iostate&, long double&) const; };
Types
char_type
Type of character the facet is instantiated on.
iter_type
Type of iterator used to scan the character buffer.
Constructor and Destructor
explicit num_get(size_t refs = 0)
Construct a num_get facet. If the refs argument is 0 then destruction of the object is delegated to the locale, or locales, containing it. This allows the user to ignore lifetime management issues. On the other had, if refs is 1 then the object must be explicitly deleted; the locale will not do so. In this case, the object can be maintained across the lifetime of multiple locales.
~num_get(); // virtual and protected
Destroy the facet
Facet ID
static locale::id id;
Unique identifier for this type of facet.
Public Member Functions
The public members of the num_get facet provide an interface to protected members. Each public member xxx has a corresponding virtual protected member do_xxx. All work is delegated to these protected members. For instance, the long version of the public get function simply calls its protected cousin do_get.
iter_type get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, bool& v) const; iter_type get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, long& v) const; iter_type get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, unsigned short& v) const; iter_type get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, unsigned int& v) const; iter_type get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, unsigned long& v) const; iter_type get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, float& v) const; iter_type get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, double& v) const; iter_type get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, long double& v) const;
Each of the eight overloads of the get function simply call the corresponding do_get function.
Protected Member Functions
virtual iter_type do_get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, bool& v) const; virtual iter_type do_get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, long& v) const; virtual iter_type do_get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, unsigned short& v) const; virtual iter_type do_get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, unsigned int& v) const; virtual iter_type do_get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, unsigned long& v) const; virtual iter_type do_get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, float& v) const; virtual iter_type do_get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& err, double& v) const; virtual iter_type do_get(iter_type in, iter_type end, ios_base& io, ios_base::iostate& long double& v) const;
The eight overloads of the do_get member function all take a sequence of characters [int,end), and extract a numeric value. The numeric value is returned in v. The io argument is used to obtain formatting information and the err argument is used to set error conditions in a calling stream.
Example
// // numget.cpp // #include <sstream> int main () { using namespace std; typedef istreambuf_iterator<char,char_traits<char> > iter_type; locale loc; ios_base::iostate state; bool bval = false; long lval = 0L; long double ldval = 0.0; iter_type end; // Get a num_get facet const num_get<char,iter_type>& tg = #ifndef _RWSTD_NO_TEMPLATE_ON_RETURN_TYPE use_facet<num_get<char,iter_type> >(loc); #else use_facet(loc,(num_get<char,iter_type>*)0); #endif { // Build an istringstream from the buffer and construct // beginning and ending iterators on it. istringstream ins("true"); iter_type begin(ins); // Get a bool value tg.get(begin,end,ins,state,bval); } cout << bval << endl; { // Get a long value istringstream ins("2422235"); iter_type begin(ins); tg.get(begin,end,ins,state,lval); } cout << lval << endl; { // Get a long double value istringstream ins("32324342.98908"); iter_type begin(ins); tg.get(begin,end,ins,state,ldval); } cout << ldval << endl; return 0; }
See Also
locale, facets, num_put, numpunct, ctype
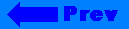
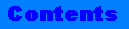
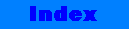
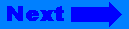
©Copyright 1996, Rogue Wave Software, Inc.