Personal tools
moneypunct, moneypunct_byname

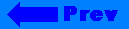
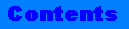
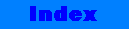
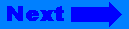
Click on the banner to return to the class reference home page.
moneypunct, moneypunct_byname
moneypunct_base moneypunct_byname
moneypunct
locale::facet
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors and Destructors
- Static Members
- Public Member Functions
- Protected Member Functions
- Example
- See Also
Summary
Monetary punctuation facets.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types |
char_type string_type |
Synopsis
#include <locale> class money_base; template <class charT, bool International = false> class moneypunct;
Description
The moneypunct facets provide formatting specifications and punctuation character for monetary values. The moneypunct facet provides punctuation based on the "C" locale, while the moneypunct_byname facet provides the same facilities for named locales.
The facet is used by money_put for outputting formatted representations of monetary values and by money_get for reading these strings back in.
money_base provides a structure, pattern, that specifies the order of syntactic elements in a monetary value and enumeration values representing those elements. The pattern struct provides a simple array of characters, field. Each index in field is taken up by an enumeration value indicating the location of a syntactic element. The enumeration values are described below:
Format Flag |
Meaning |
none |
No grouping seperator |
space |
Use space for grouping seperator |
symbol |
Currency symbol |
sign |
Sign of monetary value |
value |
The monetary value itself |
The do_pos_format an do_neg_format member functions of moneypunct both return the pattern type. See the description of these functions for further elaboration.
Interface
class money_base { public: enum part { none, space, symbol, sign, value }; struct pattern { char field[4]; }; }; template <class charT, bool International = false> class moneypunct : public locale::facet, public money_base { public: typedef charT char_type; typedef basic_string<charT> string_type; explicit moneypunct(size_t = 0); charT decimal_point() const; charT thousands_sep() const; string grouping() const; string_type curr_symbol() const; string_type positive_sign() const; string_type negative_sign() const; int frac_digits() const; pattern pos_format() const; pattern neg_format() const; static locale::id id; static const bool intl = International; protected: ~moneypunct(); // virtual virtual charT do_decimal_point() const; virtual charT do_thousands_sep() const; virtual string do_grouping() const; virtual string_type do_curr_symbol() const; virtual string_type do_positive_sign() const; virtual string_type do_negative_sign() const; virtual int do_frac_digits() const; virtual pattern do_pos_format() const; virtual pattern do_neg_format() const; }; template <class charT, bool Intl = false> class moneypunct_byname : public moneypunct<charT, Intl> { public: explicit moneypunct_byname(const char*, size_t = 0); protected: ~moneypunct_byname(); // virtual virtual charT do_decimal_point() const; virtual charT do_thousands_sep() const; virtual string do_grouping() const; virtual string_type do_curr_symbol() const; virtual string_type do_positive_sign() const; virtual string_type do_negative_sign() const; virtual int do_frac_digits() const; virtual pattern do_pos_format() const; virtual pattern do_neg_format() const; };
Types
char_type
Type of character the facet is instantiated on.
string_type
Type of character string returned by member functions.
Constructors and Destructors
explicit moneypunct(size_t refs = 0)
Constructs a moneypunct facet. If the refs argument is 0 then destruction of the object is delegated to the locale, or locales, containing it. This allows the user to ignore lifetime management issues. On the other had, if refs is 1 then the object must be explicitly deleted; the locale will not do so. In this case, the object can be maintained across the lifetime of multiple locales.
explicit moneypunct_byname(const char* name, size_t refs = 0);
Constructs a moneypunct_byname facet. Uses the named locale specified by the name argument. The refs argument serves the same purpose as it does for the moneypunct constructor.
~moneypunct(); // virtual and protected
Destroy the facet
Static Members
static locale::id id;
Unique identifier for this type of facet.
static const bool intl = Intl;
true if international representation, false otherwise.
Public Member Functions
The public members of the moneypunct and moneypunct_byname facets provide an interface to protected members. Each public member xxx has a corresponding virtual protected member do_xxx. All work is delegated to these protected members. For instance, the long version of the public decimal_point function simply calls its protected cousin do_decimal_point.
string_type curr_symbol() const; charT decimal_point() const; int frac_digits() const; string grouping() const; pattern neg_format() const; string_type negative_sign() const; pattern pos_format() const; string_type positive_sign() const; charT thousands_sep() const;
Each of these public member functions xxx simply calls the corresponding protected do_xxx function.
Protected Member Functions
virtual string_type do_curr_symbol() const;
Returns a string to use as the currency symbol.
virtual charT do_decimal_point() const;
Returns the radix separator to use if fractional digits are allowed (see do_frac_digits).
virtual int do_frac_digits() const;
Returns the number of digits in the fractional part of the monetary representation.
virtual string do_grouping() const;
Returns a string in which each character is used as an integer value to represent the number of digits in a particular grouping, starting with the rightmost group. A group is simply the digits between adjacent thousands' separators. Each group at a position larger than the size of the string gets the same value as the last element in the string. If a value is less than or equal to zero, or equal to CHAR_MAX, then the size of that group is unlimited. moneypunct returns an empty string, indicating no grouping.
virtual string_type do_negative_sign() const;
A string to use as the negative sign. The first character of this string will be placed in the position indicated by the format pattern (see do_neg_format); the rest of the characters, if any, will be placed after all other parts of the monetary value.
virtual pattern do_neg_format() const; virtual pattern do_pos_format() const;
Returns a pattern object specifying the location of the various syntactic elements in a monetary representation. The enumeration values symbol, sign, and value will appear exactly once in this pattern, with the remaining location taken by either none or space. none will never occupy the first position in the pattern and space will never occupy the first or the last position. Beyond these restrictions, elements may appear in any order. moneypunct returns {symbol, sign, none, value}.
virtual string_type do_positive_sign() const;
A string to use as the positive sign. The first character of this string will be placed in the position indicated by the format pattern (see do_pos_format); the rest of the characters, if any, will be placed after all other parts of the monetary value.
virtual charT do_thousands_sep() const;
Returns the grouping separator if grouping is allowed (see do_grouping).
Example
// // moneypun.cpp // #include <string> #include <iostream> int main () { using namespace std; locale loc; // Get a moneypunct facet const moneypunct<char,false>& mp = #ifndef _RWSTD_NO_TEMPLATE_ON_RETURN_TYPE use_facet<moneypunct<char,false> >(loc); #else use_facet(loc,(moneypunct<char,false>*)0); #endif cout << "Decimal point = " << mp.decimal_point() << endl; cout << "Thousands separator = " << mp.thousands_sep() << endl; cout << "Currency symbol = " << mp.curr_symbol() << endl; cout << "Negative Sign = " << mp.negative_sign() << endl; cout << "Digits after decimal = " << mp.frac_digits() << endl; return 0; }
See Also
locale, facets, money_put, money_get
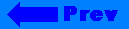
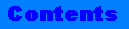
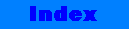
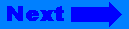
©Copyright 1996, Rogue Wave Software, Inc.