Personal tools
locale

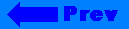
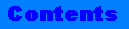
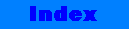
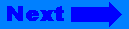
Click on the banner to return to the class reference home page.
locale
Localization Container
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors and Destructors
- Public Member Operators
- Public Member Functions
- Static Public Member Functions
- Example
- See Also
Summary
Localization class containing a polymorphic set of facets.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Member Functions |
name() operator!=() operator()() operator=() operator==() |
Synopsis
#include<locale> class locale;
Description
locale provides a localization interface and a set of indexed facets, each of which covers one particular localization issue. The default locale object is constructed on the "C" locale. Locales can also be constructed on named locales.
A calling program can determine whether a particular facet is contained in a locale by using the has_facet function, and the program can obtain a reference to that facet with the use_facet function. These are not member functions, but instead take a locale object as an argument.
locale has several important characteristics.
First, successive calls to member functions will always return the same result. This allows a calling program to safely cache the results of a call.
Any standard facet not implemented by a locale will be obtained from the global locale the first time that use_facet is called (for that facet).
Only a locale constructed from a name (i.e., "POSIX"), from parts of two named locales, or from a stream, has a name. All other locales are unnamed. Only named locales may be compared for equality. An unnamed locale is equal only to itself.
Interface
class locale { public: // types: class facet; class id; typedef int category; static const category none, collate, ctype, monetary, numeric, time, messages, all = collate | ctype | monetary | numeric | time | messages; // construct/copy/destroy: locale() throw() locale(const locale&) throw() explicit locale(const char*); locale(const locale&, const char*, category); template <class Facet> locale(const locale&, Facet*); template <class Facet> locale(const locale&, const locale&); locale(const locale&, const locale&, category); ~locale() throw(); // non-virtual const locale& operator=(const locale&) throw(); // locale operations: basic_string<char> name() const; bool operator==(const locale&) const; bool operator!=(const locale&) const; template <class charT,Traits> bool operator()(const basic_string<charT,Traits>&, const basic_string<charT,Traits>&) const; // global locale objects: static locale global(const locale&); static const locale& classic(); }; class locale::facet { protected: explicit facet(size_t refs = 0); virtual ~facet(); private: facet(const facet&); // not defined void operator=(const facet&); // not defined }; class locale::id { public: id(); private: void operator=(const id&); // not defined id(const id&); // not defined };
Types
category
Standard facets fall into eight broad categories. These are: none, collate, ctype, monetary, numeric, time, messages, and all. all is a combination of all the other categories except none. Bitwise operations may be applied to combine or screen these categories. For instance all is defined as:
(collate | ctype | monetary | numeric | time | messages)
locale member functions that take a category argument must be provided with one of the above values, or one of the constants from the old C locale (e.g., LC_CTYPE).
facet
Base class for facets. This class exists primarily to provide reference counting services to derived classes. All facets must derive from it, either directly or indirectly indirectly (e.g., facet -> ctype<char> -> my_ctype).
If the refs argument to the constructor is 0 then destruction of the object is delegated to the locale, or locales, containing it. This allows the user to ignore lifetime management issues. On the other had, if refs is 1, then the object must be explicitly deleted; the locale will not do so. In this case, the object can be maintained across the lifetime of multiple locales.
Copy construction and assignment of this class are disallowed.
id
Type used to index facets in the locale container. Every facet must contain a member of this type.
Copy construction and assignment of this type are disallowed.
Constructors and Destructors
locale() throw()
Constructs a default locale object. This locale will be the same as the last argument passed to locale::global(), or, if that function has not been called, the locale will be the same as the classic "C" locale.
locale(const locale& other) throw()
Constructs a copy of the locale argument other.
explicit locale(const char* std_name);
Constructs a locale object on the named locale indicated by std_name. Throws a runtime_error exception of if std_name is not a valid locale name.
locale(const locale& other, const char* std_name, category cat);
Construct a locale object that is a copy of other, except for the facets that are in the category specified by cat. These facets will be obtained from the named locale identified by std_name. Throws a runtime_error exception of if std_name is not a valid locale name.
Note that the resulting locale will have the same name (possibly none) as other.
template <class Facet> locale(const locale& other, Facet* f);
Constructs a locale object that is a copy of other, except for the facet of type Facet. Unless f is null, it f is used to supply the missing facet, otherwise the facet will come from other as well.
Note that the resulting locale will not have a name.
template <class Facet> locale(const locale& other, const locale& one);
Constructs a locale object that is a copy of other, except for the facet of type Facet. This missing facet is obtained from the second locale argument, one. Throws a runtime_error exception if one does not contain a facet of type Facet.
Note that the resulting locale will not have a name.
locale(const locale& other, const locale& one, category cat);
Constructs a locale object that is a copy of other, except for facets that are in the category specified by category argument cat. These missing facets are obtained from the other locale argument, one.
Note that the resulting locale will only have a name only if both other and one have names. The name will be the same as other's name.
~locale();
Destroy the locale.
Public Member Operators
const locale& operator=(const locale& other) throw();
Replaces *this with a copy of other. Returns *this.
bool operator==(const locale& other) const;
Returns true if both other and *this are the same object, if one is a copy of another, or if both have the same name. Otherwise returns false.
bool operator!=(const locale& other) const;
Returns !(*this == other)
template <class charT,Traits> bool operator()(const basic_string<charT,Traits>& s1, const basic_string<charT,Traits>& s2) const;
This operator is provided to allow a locale object to be used as a comparison object for comparing two strings. Returns the result of comparing the two strings using the compare member function of the collate<charT> facet contained in this. Specifically, this function returns the following:
use_facet< collate<charT> >(*this).compare(s1.data(), s1.data()+s1.size(), s2.data(), s2.data()+s2.size()) < 0;
This allows a locale to be used with standard algorithms, such as sort, to provide localized comparison of strings.
Public Member Functions
basic_string<char> name() const;
Returns the name of this, if this has one; otherwise returns the string "*".
Static Public Member Functions
static locale global(const locale& loc);
Sets the global locale to loc. This causes future uses of the default constructor for locale to return a copy of loc. If loc has a name, this function has the further effect of calling std::setlocale(LC_ALL,loc.name().c_str());. Returns the previous value of locale().
static const locale& classic();
Returns a locale with the same semantics as the classic "C" locale.
Example
// // locale.cpp // #include <string> #include <vector> #include <iostream> #include "codecvte.h" int main () { using namespace std; locale loc; // Default locale // Construct new locale using default locale plus // user defined codecvt facet // This facet converts from ISO Latin // Alphabet No. 1 (ISO 8859-1) to // U.S. ASCII code page 437 // This facet replaces the default for // codecvt<char,char,mbstate_t> locale my_loc(loc,new ex_codecvt); // imbue modified locale onto cout locale old = cout.imbue(my_loc); cout << "A \x93 jolly time was had by all" << endl; cout.imbue(old); cout << "A jolly time was had by all" << endl; // Create a vector of strings vector<string,allocator<void> > v; v.insert(v.begin(),"antelope"); v.insert(v.begin(),"bison"); v.insert(v.begin(),"elk"); copy(v.begin(),v.end(), ostream_iterator<string,char, char_traits<char> >(cout," ")); cout << endl; // Sort the strings using the locale as a comparitor sort(v.begin(),v.end(),loc); copy(v.begin(),v.end(), ostream_iterator<string,char, char_traits<char> >(cout," ")); cout << endl; return 0; }
See Also
facets, has_facet, use_facet, specific facet reference sections
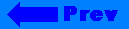
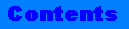
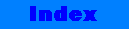
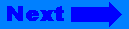
©Copyright 1996, Rogue Wave Software, Inc.