Personal tools
numpunct, numpunct_byname

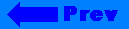
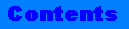
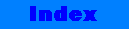
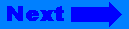
Click on the banner to return to the class reference home page.
numpunct, numpunct_byname
numpunctlocale::facet
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors and Destructors
- Facet ID
- Public Member Functions
- Protected Member Functions
- Example
- See Also
Summary
Numeric punctuation facet.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types |
char_type |
Member Functions |
decimal_point() do_decimal_point() do_falsename() do_grouping() do_thousands_sep() do_truename() falsename() grouping() thousands_sep() truename() |
Synopsis
#include <locale> template <class charT> class numpunct; template <class charT> class numpunct_byname;
Description
The numpunct<charT> facet specifies numeric punctuation. This template provides punctuation based on the "C" locale, while the numpunct_byname facet provides the same facilities for named locales.
Both num_put and num_get make use of this facet.
Interface
template <class charT> class numpunct : public locale::facet { public: typedef charT char_type; typedef basic_string<charT> string_type; explicit numpunct(size_t refs = 0); char_type decimal_point() const; char_type thousands_sep() const; string grouping() const; string_type truename() const; string_type falsename() const; static locale::id id; protected: ~numpunct(); // virtual virtual char_type do_decimal_point() const; virtual char_type do_thousands_sep() const; virtual string do_grouping() const; virtual string_type do_truename() const; // for bool virtual string_type do_falsename() const; // for bool }; template <class charT> class numpunct_byname : public numpunct<charT> { public: explicit numpunct_byname(const char*, size_t refs = 0); protected: ~numpunct_byname(); // virtual virtual char_type do_decimal_point() const; virtual char_type do_thousands_sep() const; virtual string do_grouping() const; virtual string_type do_truename() const; // for bool virtual string_type do_falsename() const; // for bool };
Types
char_type
Type of character upon which the facet is instantiated.
string_type
Type of character string returned by member functions.
Constructors and Destructors
explicit numpunct(size_t refs = 0)
Construct a numpunct facet. If the refs argument is 0 then destruction of the object is delegated to the locale, or locales, containing it. This allows the user to ignore lifetime management issues. On the other had, if refs is 1 then the object must be explicitly deleted; the locale will not do so. In this case, the object can be maintained across the lifetime of multiple locales.
explicit numpunct_byname(const char* name, size_t refs = 0);
Construct a numpunct_byname facet. Use the named locale specified by the name argument. The refs argument serves the same purpose as it does for the numpunct constructor.
~numpunct(); // virtual and protected ~numpunct_byname(); // virtual and protected
Destroy the facet
Facet ID
static locale::id id;
Unique identifier for this type of facet.
Public Member Functions
The public members of the numpunct facet provide an interface to protected members. Each public member xxx has a corresponding virtual protected member do_xxx. All work is delegated to these protected members. For instance, the long version of the public grouping function simply calls its protected cousin do_grouping.
char_type decimal_point() const; string_type falsename() const; string grouping() const; char_type thousands_sep() const; string_type truename() const;
Each of these public member functions xxx simply call the corresponding protected do_xxx function.
Protected Member Functions
virtual char_type do_decimal_point() const;
Returns the decimal radix separator . numpunct returns '.'.
virtual string_type do_falsename() const; // for bool virtual string_type do_truename() const; // for bool
Returns a string representing the name of the boolean values true and false respectively . numpunct returns "true" and "false".
virtual string do_grouping() const;
Returns a string in which each character is used as an integer value to represent the number of digits in a particular grouping, starting with the rightmost group. A group is simply the digits between adjacent thousands separators. Each group at a position larger than the size of the string gets the same value as the last element in the string. If a value is less than or equal to zero, or equal to CHAR_MAX then the size of that group is unlimited. numpunct returns an empty string, indicating no grouping.
virtual char_type do_thousands_sep() const;
Returns the decimal digit group separator. numpunct returns ','.
Example
// // numpunct.cpp // #include <iostream> int main () { using namespace std; locale loc; // Get a numpunct facet const numpunct<char>& np = #ifndef _RWSTD_NO_TEMPLATE_ON_RETURN_TYPE use_facet<numpunct<char> >(loc); #else use_facet(loc,(numpunct<char>*)0); #endif cout << "Decimal point = " << np.decimal_point() << endl; cout << "Thousands separator = " << np.thousands_sep() << endl; cout << "True name = " << np.truename() << endl; cout << "False name = " << np.falsename() << endl; return 0; }
See Also
locale, facets, num_put, num_get, ctype
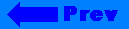
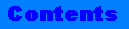
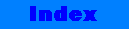
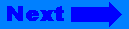
©Copyright 1996, Rogue Wave Software, Inc.