Personal tools
ctype

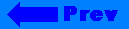
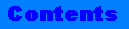
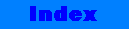
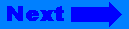
Click on the banner to return to the class reference home page.
ctype
ctype_base ctype...
locale::facet
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Specialization
- Description
- Interface
- Type
- Constructor and Destructor
- Public Member Functions
- Facet ID
- Protected Member Functions
- Example
- See Also
Summary
A facet that provides character classification facilities.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types |
id |
Member Functions | |
char_type do_is() do_narrow() do_scan_is() do_scan_not() do_tolower() do_toupper() do_widen() is() narrow() |
scan_is() scan_not() tolower() toupper() widen() |
Synopsis
#include <locale> class ctype_base; template <class charT> class ctype;
Specialization
class ctype<char>;
Description
ctype<charT> is the character classification facet. This facet provides facilities for classifying characters and performing simple conversions. ctype<charT> provides conversions for upper to lower and lower to upper case. The facet also provides conversions between charT, and char. ctype<charT> relies on ctype_base for a set of masks that identify the various classes of characters. These classes are:
-
space
cntrl
upper
lower
alpha
digit
punct
xdigit
alnum
graph
The masks are passed to member functions of ctype in order to obtain verify the classifications of a character or range of characters.
Interface
class ctype_base { public: enum mask { space, print, cntrl, upper, lower, alpha, digit, punct, xdigit, alnum=alpha|digit, graph=alnum|punct }; }; template <class charT> class ctype : public locale::facet, public ctype_base { public: typedef charT char_type; explicit ctype(size_t); bool is(mask, charT) const; const charT* is(const charT*, const charT*, mask*) const; const charT* scan_is(mask, const charT*, const charT*) const; const charT* scan_not(mask, const charT*, const charT*) const; charT toupper(charT) const; const charT* toupper(charT*, const charT*) const; charT tolower(charT) const; const charT* tolower(charT*, const charT*) const; charT widen(char) const; const char* widen(const char*, const char*, charT*) const; char narrow(charT, char) const; const charT* narrow(const charT*, const charT*, char, char*) const; static locale::id id; protected: ~ctype(); // virtual virtual bool do_is(mask, charT) const; virtual const charT* do_is(const charT*, const charT*, mask*) const; virtual const charT* do_scan_is(mask, const charT*, const charT*) const; virtual const charT* do_scan_not(mask, const charT*, const charT*) const; virtual charT do_toupper(charT) const; virtual const charT* do_toupper(charT*, const charT*) const; virtual charT do_tolower(charT) const; virtual const charT* do_tolower(charT*, const charT*) const; virtual charT do_widen(char) const; virtual const char* do_widen(const char*, const char*, charT*) const; virtual char do_narrow(charT, char) const; virtual const charT* do_narrow(const charT*, const charT*, char, char*) const; };
Type
char_type
Type of character the facet is instantiated on.
Constructor and Destructor
explicit ctype(size_t refs = 0)
Construct a ctype facet. If the refs argument is 0 then destruction of the object is delegated to the locale, or locales, containing it. This allows the user to ignore lifetime management issues. On the other had, if refs is 1 then the object must be explicitly deleted; the locale will not do so. In this case, the object can be maintained across the lifetime of multiple locales.
~ctype(); // virtual and protected
Destroy the facet.
Public Member Functions
The public members of the ctype facet provide an interface to protected members. Each public member xxx has a corresponding virtual protected member do_xxx. All work is delegated to these protected members. For instance, the public widen function simply calls its protected cousin do_widen.
bool is(mask m, charT c) const; const charT* is(const charT* low, const charT* high, mask* vec) const;
Returns do_is(m,c) or do_is(low,high,vec).
char narrow(charT c, char dfault) const; const charT* narrow(const charT* low, const charT*, char dfault, char* to) const;
Returns do_narrow(c,dfault) or do_narrow(low,high,dfault,to).
const charT* scan_is(mask m, const charT*, const charT* high) const;
Returns do_scan_is(m,low,high).
const charT* scan_not(mask m, const charT* low, const charT* high) const;
Returns do_scan_not(m,low,high).
charT tolower(charT c) const; const charT* tolower(charT* low, const charT* high) const;
Returns do_tolower(c) or do_tolower(low,high).
charT toupper(charT) const; const charT* toupper(charT* low, const charT* high) const;
Returns do_toupper(c) or do_toupper(low,high).
charT widen(char c) const; const char* widen(const char* low, const char* high, charT* to) const;
Returns do_widen(c) or do_widen(low,high,to).
Facet ID
static locale::id id;
Unique identifier for this type of facet.
Protected Member Functions
virtual bool do_is(mask m, charT c) const;
Returns true if c matches the classification indicated by the mask m, where m is one of the values available from ctype_base. For instance, the following call returns true since 'a' is an alphabetic character:
ctype<char>().is(ctype_base::alpha,'a');
See ctype_base for a description of the masks.
virtual const charT* do_is(const charT* low, const charT* high, mask* vec) const;
Fills vec with every mask from ctype_base that applies to the range of characters indicated by [low,high). See ctype_base for a description of the masks. For instance, after the following call v would contain {alpha, lower, print,alnum ,graph}:
char a[] = "abcde"; ctype_base::mask v[12]; ctype<char>().is(a,a+5,v);
This function returns high.
virtual char do_narrow(charT, char dfault) const;
Returns the appropriate char representation for c, if such exists. Otherwise do_narrow returns dfault.
virtual const charT* do_narrow(const charT* low, const charT* high, char dfault, char* dest) const;
Converts each character in the range [low,high) to its char representation, if such exists. If a char representation is not available then the character will be converted to dfault. Returns high.
virtual const charT* do_scan_is(mask m, const charT* low, const charT* high) const;
Finds the first character in the range [low,high) that matches the classification indicated by the mask m.
virtual const charT* do_scan_not(mask m, const charT* low, const charT* high) const;
Finds the first character in the range [low,high) that does not match the classification indicated by the mask m.
virtual charT do_tolower(charT) const;
Returns the lower case representation of c, if such exists, otherwise returns c;
virtual const charT* do_tolower(charT* low, const charT* high) const;
Converts each character in the range [low,high) to its lower case representation, if such exists. If a lower case representation does not exist then the character is not changed. Returns high.
virtual charT do_toupper(charT c) const;
Returns the upper case representation of c, if such exists, otherwise returns c;
virtual const charT* do_toupper(charT* low, const charT* high) const;
Converts each character in the range [low,high) to its upper case representation, if such exists. If an upper case representation does not exist then the character is not changed. Returns high.
virtual charT do_widen(char c) const;
Returns the appropriate charT representation for c.
virtual const char* do_widen(const char* low, const char* high,charT* dest) const;
Converts each character in the range [low,high) to its charT representation. Returns high.
Example
// // ctype.cpp // #include <iostream> int main () { using namespace std; locale loc; string s1("blues Power"); // Get a reference to the ctype<char> facet const ctype<char>& ct = #ifndef _RWSTD_NO_TEMPLATE_ON_RETURN_TYPE use_facet<ctype<char> >(loc); #else use_facet(loc,(ctype<char>*)0); #endif // Check the classification of the 'a' character cout << ct.is(ctype_base::alpha,'a') << endl; cout << ct.is(ctype_base::punct,'a') << endl; // Scan for the first upper case character cout << (char)*(ct.scan_is(ctype_base::upper, s1.begin(),s1.end())) << endl; // Convert characters to upper case ct.toupper(s1.begin(),s1.end()); cout << s1 << endl; return 0; }
See Also
locale, facets, collate, ctype<char>, ctype_byname
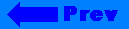
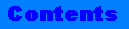
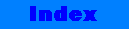
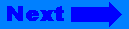
©Copyright 1996, Rogue Wave Software, Inc.