Personal tools
collate, collate_byname

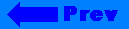
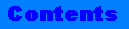
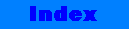
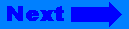
Click on the banner to return to the class reference home page.
collate, collate_byname
collate_bynamecollate
locale::facet
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors and Destructors
- Facet ID
- Public Member Functions
- Protected Member Functions
- Example
- See Also
Summary
String collation, comparison, and hashing facet.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types |
char_type id string_type |
Member Functions |
compare() do_compare() do_hash() do_transform() hash() transform() |
Synopsis
#include <locale> template <class charT> class collate; template <class charT> class collate_byname;
Description
The collate and collate_byname facets provides string collation, comparison, and hashing facilities. collate provides these facilities for the "C" locale, while collate_byname provides the same thing for named locales.
Interface
template <class charT> class collate : public locale::facet { public: typedef charT char_type; typedef basic_string<charT> string_type; explicit collate(size_t refs = 0); int compare(const charT*, const charT*, const charT*, const charT*) const; string_type transform(const charT*, const charT*) const; long hash(const charT*, const charT*) const; static locale::id id; protected: ~collate(); // virtual virtual int do_compare(const charT*, const charT*, const charT*, const charT*) const; virtual string_type do_transform(const charT*, const charT*) const; virtual long do_hash (const charT*, const charT*) const; }; template <class charT> class collate_byname : public collate<charT> { public: explicit collate_byname(const char*, size_t = 0); protected: ~collate_byname(); // virtual virtual int do_compare(const charT*, const charT*, const charT*, const charT*) const; virtual string_type do_transform(const charT*, const charT*) const; virtual long do_hash(const charT*, const charT*) const; };
Types
char_type
Type of character the facet is instantiated on.
string_type
Type of character string returned by member functions.
Constructors and Destructors
explicit collate(size_t refs = 0)
Construct a collate facet. If the refs argument is 0, destruction of the object is delegated to the locale, or locales, containing it. This allows the user to ignore lifetime management issues. On the other had, if refs is 1, the object must be explicitly deleted: the locale will not do so. In this case, the object can be maintained across the lifetime of multiple locales.
explicit collate_byname(const char* name, size_t refs = 0);
Construct a collate_byname facet. Use the named locale specified by the name argument. The refs argument serves the same purpose as it does for the collate constructor.
~collate(); // virtual and protected ~collate_byname(); // virtual and protected
Destroy the facet
Facet ID
static locale::id id;
Unique identifier for this type of facet.
Public Member Functions
The public members of the collate facet provide an interface to protected members. Each public member xxx has a corresponding virtual protected member do_xxx. All work is delegated to these protected members. For instance, the long version of the public grouping function simply calls its protected cousin do_grouping.
int compare(const charT* low1, const charT* high1, const charT* low2, const charT* high2) const; long hash(const charT* low, const charT* high) const; string_type transform(const charT* low, const charT* high) const;
Each of these public member functions xxx simply call the corresponding protected do_xxx function.
Protected Member Functions
virtual int do_compare(const charT* low1, const charT* high1, const charT* low2, const charT* high2) const;
Returns 1 if the character string represented by the range [low1,high1) is greater than the character string represented by the range [low2,high2), -1 if first string is less than the second, or 0 if the two are equal. collate uses a lexicographical comparison.
virtual long do_hash( const charT* low, const charT* high)
Generate a has value from a string defined by the range of characters [low,high). Given two strings that compare equal (i.e. do_compare returns 0), do_hash returns an integer value that is the same for both strings. For differing strings the probability that the return value will be equal is approximately 1.0/numeric_limits<unsigned long>::max().
virtual string_type do_transform(const charT* low, const charT* high) const;
Returns a string that will yield the same result in a lexicographical comparison with another string returned from transform as does the do_compare function applied to the original strings. In other words, the result of applying a lexicographical comparison to two strings returned from transform will be the same as applying do_compare to the original strings passed to transform.
Example
// // collate.cpp // #include <iostream> int main () { using namespace std; locale loc; string s1("blue"); string s2("blues"); // Get a reference to the collate<char> facet const collate<char>& co = #ifndef _RWSTD_NO_TEMPLATE_ON_RETURN_TYPE use_facet<collate<char> >(loc); #else use_facet(loc,(collate<char>*)0); #endif // Compare two strings cout << co.compare(s1.begin(),s1.end(), s2.begin(),s2.end()-1) << endl; cout << co.compare(s1.begin(),s1.end(), s2.begin(),s2.end()) << endl; // Retrieve hash values for two strings cout << co.hash(s1.begin(),s1.end()) << endl; cout << co.hash(s2.begin(),s2.end()) << endl; return 0; }
See Also
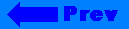
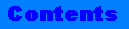
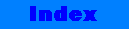
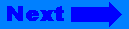
©Copyright 1996, Rogue Wave Software, Inc.