Personal tools
basic_istream

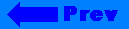
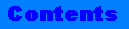
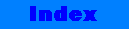
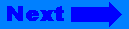
Click on the banner to return to the class reference home page.
basic_istream
basic_istreambasic_ios
ios_base
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Public Constructor
- Public Destructor
- Sentry Class
- Extractors
- Unformatted Functions
- Non Member Functions
- Examples
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | ||
char_type int_type ios_type istream |
istream_type off_type pos_type streambuf_type |
traits_type wistream |
Member Functions | |
bool() gcount() get() getline() ignore() >()">operator>>() peek() putback() read() readsome() |
seekg() sentry() sync() tellg() unget() ws() ~sentry() |
Synopsis
#include <istream> template<class charT, class traits = char_traits<charT> > class basic_istream : virtual public basic_ios<charT, traits>
Description
The class basic_istream defines a number of member function signatures that assist in reading and interpreting input from sequences controlled by a stream buffer.
Two groups of member function signatures share common properties: the formatted input functions (or extractors) and the unformatted input functions. Both groups of input functions obtain (or extract) input characters by calling basic_streambuf member functions. They both begin by constructing an object of class basic_istream::sentry and, if this object is in good state after construction, the function endeavors to obtain the requested input. The sentry object performs exception safe initialization, such as controlling the status of the stream or locking it in multithread environment.
Some formatted input functions parse characters extracted from the input sequence, converting the result to a value of some scalar data type, and storing the converted value in an object of that scalar type. The conversion behavior is locale dependent, and directly depend on the locale object imbued in the stream.
Interface
template<class charT, class traits = char_traits<charT> > class basic_istream : virtual public basic_ios<charT, traits> { public: typedef basic_istream<charT, traits> istream_type; typedef basic_ios<charT, traits> ios_type; typedef basic_streambuf<charT, traits> streambuf_type; typedef traits traits_type; typedef charT char_type; typedef typename traits::int_type int_type; typedef typename traits::pos_type pos_type; typedef typename traits::off_type off_type; explicit basic_istream(basic_streambuf<charT, traits> *sb); virtual ~basic_istream(); class sentry { public: inline explicit sentry(basic_istream<charT,traits>&, bool noskipws = 0); ~sentry(); operator bool (); }; istream_type& operator>>(istream_type& (*pf)(istream_type&)); istream_type& operator>>(ios_base& (*pf)(ios_base&)); istream_type& operator>>(ios_type& (*pf)(ios_type&)); istream_type& operator>>(bool& n); istream_type& operator>>(short& n); istream_type& operator>>(unsigned short& n); istream_type& operator>>(int& n); istream_type& operator>>(unsigned int& n); istream_type& operator>>(long& n); istream_type& operator>>(unsigned long& n); istream_type& operator>>(float& f); istream_type& operator>>(double& f); istream_type& operator>>(long double& f); istream_type& operator>>(void*& p); istream_type& operator>>(streambuf_type& sb); istream_type& operator>>(streambuf_type *sb); int_type get(); istream_type& get(char_type *s, streamsize n, char_type delim); istream_type& get(char_type *s, streamsize n); istream_type& get(char_type& c); istream_type& get(streambuf_type& sb,char_type delim); istream_type& get(streambuf_type& sb); istream_type& getline(char_type *s, streamsize n,char_type delim); istream_type& getline(char_type *s, streamsize n); istream_type& ignore(streamsize n = 1, int_type delim = traits::eof()); istream_type& read(char_type *s, streamsize n); streamsize readsome(char_type *s, streamsize n); int peek(); pos_type tellg(); istream_type& seekg(pos_type&); istream_type& seekg(off_type&, ios_base::seekdir); istream_type& putback(char_type c); istream_type& unget(); streamsize gcount() const; int sync(); protected: explicit basic_istream( ); }; //global function template<class charT, class traits> basic_istream<charT, traits>& ws(basic_istream<charT, traits>&); template<class charT, class traits> basic_istream<charT, traits>& operator>> (basic_istream<charT, traits>&, charT&); template<class charT, class traits> basic_istream<charT, traits>& operator>> (basic_istream<charT, traits>&, charT*); template<class traits> basic_istream<char, traits>& operator>> (basic_istream<char, traits>&, unsigned char&); template<class traits> basic_istream<char, traits>& operator>> (basic_istream<char, traits>&, signed char&); template<class traits> basic_istream<char, traits>& operator>> (basic_istream<char, traits>&, unsigned char*); template<class traits> basic_istream<char, traits>& operator>> (basic_istream<char, traits>&, signed char*);
Types
char_type
The type char_type is a synonym for them template parameter charT.
int_type
The type int_type is a synonym of type traits::in_type.
ios_type
The type ios_type is a synonym for basic_ios<charT, traits> .
istream
The type istream is an instantiation of class basic_istream on type char:
typedef basic_istream<char> istream;
istream_type
The type istream_type is a synonym for basic_istream<charT, traits>.
off_type
The type off_type is a synonym of type traits::off_type.
pos_type
The type pos_type is a synonym of type traits::pos_type.
streambuf_type
The type streambuf_type is a synonym for basic_streambuf<charT, traits> .
traits_type
The type traits_type is a synonym for the template parameter traits.
wistream
The type wistream is an instantiation of class basic_istream on type wchar_t:
typedef basic_istream<wchar_t> wistream;
Public Constructor
explicit basic_istream(basic_streambuf<charT, traits>* sb);
Constructs an object of class basic_istream, assigning initial values to the base class by calling basic_ios::init(sb).
Public Destructor
virtual ~basic_istream();
Destroys an object of class basic_istream.
Sentry Class
explicit sentry(basic_istream<charT,traits>&, bool noskipws=0);
Prepares for formatted or unformatted input. First if the basic_ios member function tie() is not a null pointer, the function synchronizes the output sequence with any associated stream. If noskipws is zero and the ios_base member function flags() & skipws is nonzero, the function extracts and discards each character as long as the next available input character is a white space character. If after any preparation is completed the basic_ios member function good() is true, the sentry conversion function operator bool() will return true. Otherwise it will return false. In multithread environment the sentry object constructor is responsible for locking the stream and the stream buffer associated with the stream.
~sentry();
Destroys an object of class sentry. In multithread environment, the sentry object destructor is responsible for unlocking the stream and the stream buffer associated with the stream.
operator bool();
If after any preparation is completed the basic_ios member function good() is true, the sentry conversion function operator bool() will return true else it will return false.
Extractors
>()">istream_type& operator>>(istream_type& (*pf) (istream_type&));
Calls pf(*this), then return *this. See, for example, the function signature ws(basic_istream&).
istream_type& operator>>(ios_type& (*pf) (ios_type&));
Calls pf(*this), then return *this.
istream_type& operator>>(ios_base& (*pf) (ios_base&));
Calls pf(*this), then return *this. See, for example, the function signature dec(ios_base&).
istream_type& operator>>(bool& n);
Converts a Boolean value, if one is available, and stores it in n. If the ios_base member function flag() & ios_base::boolalpha is false it tries to read an integer value, which if found must be 0 or 1. If the boolalpha flag is true, it reads characters until it determines whether the characters read are correct according to the locale function numpunct<>::truename() or numpunct<>::falsename(). If no match is found, it calls the basic_ios member function setstate(failbit), which may throw ios_base::failure.
istream_type& operator>>(short& n);
Converts a signed short integer, if one is available, and stores it in n, then returns *this.
istream_type& operator>>(unsigned short& n);
Converts an unsigned short integer, if one is available, and stores it in n, then return *this.
istream_type& operator>>(int& n);
Converts a signed integer, if one is available, and stores it in n, then return *this.
istream_type& operator>>(unsigned int& n);
Converts an unsigned integer, if one is available, and stores it in n, then return *this.
istream_type& operator>>(long& n);
Converts a signed long integer, if one is available, and stores it in n, then returns *this.
istream_type& operator>>(unsigned long& n);
Converts an unsigned long integer, if one is available, and stores it in n, then returns *this.
istream_type& operator>>(float& f);
Converts a float, if one is available, and stores it in f, then returns *this.
istream_type& operator>>(double& f);
Converts a double, if one is available, and stores it in f, then returns *this.
istream_type& operator>>(long double& f);
Converts a long double, if one is available, and stores it in f, then returns *this.
istream_type& operator>>(void*& p);
Extracts a void pointer, if one is available, and stores it in p, then return *this.
istream_type& operator>>(streambuf_type* sb);
end-of-file occurs on the input sequence
inserting in the output sequence fails
an exception occurs
If sb is null, calls the basic_ios member function setstate(badbit), which may throw ios_base::failure. Otherwise extracts characters from *this and inserts them in the output sequence controlled by sb. Characters are extracted and inserted until any of the following occurs:
If the function stores no characters, it calls the basic_ios member function setstate(failbit), which may throw ios_base::failure. If failure was due to catching an exception thrown while extracting characters from sb and failbit is on in exception(), then the caught exception is rethrown.
istream_type& operator>>(streambuf_type& sb);
end-of-file occurs on the input sequence
inserting in the output sequence fails
an exception occurs
Extracts characters from *this and inserts them in the output sequence controlled by sb. Characters are extracted and inserted until any of the following occurs:
If the function stores no characters, it calls the basic_ios member function setstate(failbit), which may throw ios_base::failure. If failure was due to catching an exception thrown while extracting characters from sb and failbit is on in exception(), then the caught exception is rethrown.
Unformatted Functions
streamsize gcount() const;
Returns the number of characters extracted by the last unformatted input member function called.
int_type get();
Extracts a character, if one is available. Otherwise, the function calls the basic_ios member function setstate(failbit), which may throw ios_base::failure. Returns the character extracted or traits::eof() if none is available.
istream_type& get(char_type& c);
Extracts a character, if one is available, and assigns it to c. Otherwise, the function calls the basic_ios member function setstate(failbit), which may throw ios_base::failure.
istream_type& get(char_type* s, streamsize n,char_type delim);
n-1 characters are stored
end-of-file occurs on the input sequence
the next available input character == delim.
Extracts characters and stores them into successive locations of an array whose first element is designated by s. Characters are extracted and stored until any of the following occurs:
If the function stores no characters, it calls the basic_ios member function setstate(failbit), which may throw ios_base::failure. In any case, it stores a null character into the next successive location of the array.
istream_type& get(char_type* s, streamsize n);
Calls get(s,n,widen('\n')).
istream_type& get(streambuf_type& sb,char_type delim);
end-of-file occurs on the input sequence
inserting in the output sequence fails
the next available input character == delim.
an exception occurs
Extracts characters and inserts them in the output sequence controlled by sb. Characters are extracted and inserted until any of the following occurs:
If the function stores no characters, it calls the basic_ios member function setstate(failbit), which may throw ios_base::failure. If failure was due to catching an exception thrown while extracting characters from sb and failbit is on in exception(), then the caught exception is rethrown.
istream_type& get(streambuf_type& sb);
Calls get(sb,widen('\n')).
istream_type& getline(char_type* s, streamsize n, char_type delim);
n-1 characters are stored
end-of-file occurs on the input sequence
the next available input character == delim.
Extracts characters and stores them into successive locations of an array whose first element is designated by s. Characters are extracted and stored until any of the following occurs:
If the function stores no characters, it calls the basic_ios member function setstate(failbit), which may throw ios_base::failure. In any case, it stores a null character into the next successive location of the array.
istream_type& getline(char_type* s, streamsize n);
Calls getline(s,n,widen('\n')).
istream_type& ignore(streamsize n=1, int_type delim=traits::eof());
n characters are extracted
end-of-file occurs on the input sequence
the next available input character == delim.
Extracts characters and discards them. Characters are extracted until any of the following occurs:
int_type peek();
Returns traits::eof() if the basic_ios member function good() returns false. Otherwise, returns the next available character. Does not increment the current get pointer.
istream_type& putback(char_type c);
Insert c in the putback sequence.
istream_type& read(char_type* s, streamsize n);
n characters are stored
end-of-file occurs on the input sequence
Extracts characters and stores them into successive locations of an array whose first element is designated by s. Characters are extracted and stored until any of the following occurs:
If the function does not store n characters, it calls the basic_ios member function setstate(failbit), which may throw ios_base::failure.
streamsize readsome(char_type* s, streamsize n);
If rdbuf()->in_avail() == 0, extracts no characters
If rdbuf()->in_avail() > 0, extracts min( rdbuf()->in_avail(), n)
Extracts characters and stores them into successive locations of an array whose first element is designated by s. If rdbuf()->in_avail() == -1, calls the basic_ios member function setstate(eofbit).
In any case the function returns the number of characters extracted.
istream_type& seekg(pos_type& pos);
If the basic_ios member function fail() returns false, executes rdbuf()->pubseekpos(pos), which will position the current pointer of the input sequence at the position designated by pos.
istream_type& seekg(off_type& off, ios_base::seekdir dir);
If the basic_ios member function fail() returns false, executes rdbuf()->pubseekpos(off,dir), which will position the current pointer of the input sequence at the position designated by off and dir.
int sync();
If rdbuf() is a null pointer, return -1. Otherwise, calls rdbuf()->pubsync() and if that function returns -1 calls the basic_ios member function setstate(badbit). The purpose of this function is to synchronize the internal input buffer, with the external sequence of characters.
pos_type tellg();
If the basic_ios member function fail() returns true, tellg() returns pos_type(off_type(-1)) to indicate failure. Otherwise it returns the current position of the input sequence by calling rdbuf()->pubseekoff(0,cur,in).
istream_type& unget();
If rdbuf() is not null, calls rdbuf()->sungetc(). If rdbuf() is null or if sungetc() returns traits::eof(), calls the basic_ios member function setstate(badbit).
Non Member Functions
template<class charT, class traits> basic_istream<charT, traits>& operator>>(basic_istream<charT, traits>& is, charT& c);
Extracts a character if one is available, and stores it in c. Otherwise the function calls the basic_ios member function setstate(failbit), which may throw ios_base::failure.
template<class charT, class traits> basic_istream<charT, traits>& operator>>(basic_istream<charT, traits>& is, charT* s);
if is.witdh()>0, is.witdh()-1 characters are extracted
end-of-file occurs on the input sequence
the next available input character is a white space.
Extracts characters and stores them into successive locations of an array whose first element is designated by s. If the ios_base member function is.width() is greater than zero, then is.width() is the maximum number of characters stored. Characters are extracted and stored until any of the following occurs:
If the function stores no characters, it calls the basic_ios member function setstate(failbit), which may throw ios_base::failure. In any case, it then stores a null character into the next successive location of the array and calls width(0).
Template<class traits> basic_istream<char, traits>& operator>>(basic_istream<char, traits>& is, unsigned char& c);
Returns is >> (char&)c.
Template<class traits> basic_istream<char, traits>& operator>>(basic_istream<char, traits>& is, signed char& c);
Returns is >> (char&)c.
Template<class traits> basic_istream<char, traits>& operator>>(basic_istream<char, traits>& is, unsigned char* c);
Returns is >> (char*)c.
Template<class traits> basic_istream<char, traits>& operator>>(basic_istream<char, traits>& is, signed char* c);
Returns is >> (char*)c.
template<class charT, class traits> basic_istream<charT, traits>& ws(basic_istream<charT, traits>& is);
Skips any white space in the input sequence and returns is.
Examples
// // stdlib/examples/manual/istream1.cpp // #include<iostream> #include<istream> #include<fstream> void main ( ) { using namespace std; float f= 3.14159; int i= 3; char s[200]; // open a file for read and write operations ofstream out("example", ios_base::in | ios_base::out | ios_base::trunc); // tie the istream object to the ofstream filebuf istream in (out.rdbuf()); // output to the file out << "Annie is the Queen of porting" << endl; out << f << endl; out << i << endl; // seek to the beginning of the file in.seekg(0); f = i = 0; // read from the file using formatted functions in >> s >> f >> i; // seek to the beginning of the file in.seekg(0,ios_base::beg); // output the all file to the standard output cout << in.rdbuf(); // seek to the beginning of the file in.seekg(0); // read the first line in the file // "Annie is the Queen of porting" in.getline(s,100); cout << s << endl; // read the second line in the file // 3.14159 in.getline(s,100); cout << s << endl; // seek to the beginning of the file in.seekg(0); // read the first line in the file // "Annie is the Queen of porting" in.get(s,100); // remove the newline character in.ignore(); cout << s << endl; // read the second line in the file // 3.14159 in.get(s,100); cout << s << endl; // remove the newline character in.ignore(); // store the current file position istream::pos_type position = in.tellg(); out << "replace the int" << endl; // move back to the previous saved position in.seekg(position); // output the remain of the file // "replace the int" // this is equivalent to // cout << in.rdbuf(); while( !char_traits<char>::eq_int_type(in.peek(), char_traits<char>::eof()) ) cout << char_traits<char>::to_char_type(in.get()); cout << "\n\n\n" << flush; } // // istream example two // #include <iostream> void main ( ) { using namespace std; char p[50]; // remove all the white spaces cin >> ws; // read characters from stdin until a newline // or 49 characters have been read cin.getline(p,50); // output the result to stdout cout << p; }
See Also
char_traits(3C++), ios_base(3C++), basic_ios(3C++), basic_streambuf(3C++), basic_iostream(3C++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Section 27.6.1
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
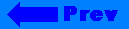
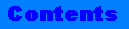
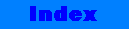
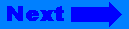
©Copyright 1996, Rogue Wave Software, Inc.