Personal tools
ios_base

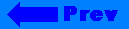
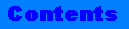
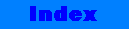
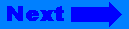
Click on the banner to return to the class reference home page.
ios_base
Base Class
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Public Constructor
- Public Destructor
- Public Member Functions
- The Class failure
- Class Init
- Non-Member Functions
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | |
event_callback fmtflags iostate openmode |
seekdir |
Synopsis
#include <ios> class ios_base;
Description
The class ios_base defines several member types:
A class failure derived from exception.
A class Init.
Three bitmask types, fmtflags, iostate and openmode.
Two enumerated types, seekdir, and event.
It maintains several kinds of data:
Control information that influences how to interpret (format) input sequences and how to generate (format) output sequences.
Locale object used within the stream classes.
Additional information that is stored by the program for its private use.
Interface
class ios_base { public: class failure : public exception { public: explicit failure(const string& msg); virtual ~failure() throw(); virtual const char* what() const throw(); }; typedef int iostate; enum io_state { goodbit = 0x00, badbit = 0x01, eofbit = 0x02, failbit = 0x04 }; typedef int openmode; enum open_mode { app = 0x01, binary = 0x02, in = 0x04, out = 0x08, trunc = 0x10, ate = 0x20 }; typedef int seekdir; enum seek_dir { beg = 0x0, cur = 0x1, end = 0x2 }; typedef int fmtflags; enum fmt_flags { boolalpha = 0x0001, dec = 0x0002, fixed = 0x0004, hex = 0x0008, internal = 0x0010, left = 0x0020, oct = 0x0040, right = 0x0080, scientific = 0x0100, showbase = 0x0200, showpoint = 0x0400, showpos = 0x0800, skipws = 0x1000, unitbuf = 0x2000, uppercase = 0x4000, adjustfield = left | right | internal, basefield = dec | oct | hex, floatfield = scientific | fixed }; enum event { erase_event = 0x0001, imbue_event = 0x0002, copyfmt_event = 0x004 }; typedef void (*event_callback) (event, ios_base&, int index); void register_callback(event_callback fn, int index); class Init; fmtflags flags() const; fmtflags flags(fmtflags fmtfl); fmtflags setf(fmtflags fmtfl); fmtflags setf(fmtflags fmtfl, fmtflags mask); void unsetf(fmtflags mask); ios_base& copyfmt(const ios_base& rhs); streamsize precision() const; streamsize precision(streamsize prec); streamsize width() const; streamsize width(streamsize wide); locale imbue(const locale& loc); locale getloc() const static int xalloc(); long& iword(int index); void*& pword(int index); bool synch_with_stdio(bool sync = true); bool is_synch(); protected: ios_base(); ~ios_base(); private: union ios_user_union { long lword; void* pword; }; union ios_user_union *userwords_; }; ios_base& boolalpha(ios_base&); ios_base& noboolalpha(ios_base&); ios_base& showbase(ios_base&); ios_base& noshowbase(ios_base&); ios_base& showpoint(ios_base&); ios_base& noshowpoint(ios_base&); ios_base& showpos(ios_base&); ios_base& noshowpos(ios_base&); ios_base& skipws(ios_base&); ios_base& noskipws(ios_base&); ios_base& uppercase(ios_base&); ios_base& nouppercase(ios_base&); ios_base& internal(ios_base&); ios_base& left(ios_base&); ios_base& right(ios_base&); ios_base& dec(ios_base&); ios_base& hex(ios_base&); ios_base& oct(ios_base&); ios_base& fixed(ios_base&); ios_base& scientific(ios_base&); ios_base& unitbuf(ios_base&); ios_base& nounitbuf(ios_base&);
Types
fmtflags
The type fmtflags is a bitmask type. Setting its elements has the following effects:
showpos |
Generates a + sign in non-negative generated numeric output. |
showbase |
Generates a prefix indicating the numeric base of generated integer output. |
uppercase |
Replaces certain lowercase letters with their uppercase equivalents in generated output. |
showpoint |
Generates a decimal-point character unconditionally in generated floating-point output. |
boolalpha |
Inserts and extracts bool type in alphabetic format. |
unitbuf |
Flushes output after each output operation. |
internal |
Adds fill characters at a designated internal point in certain generated output, or identical to right if no such point is designated. |
left |
Adds fill characters on the right (final positions) of certain generated output. |
right |
Adds fill characters on the left (initial positions) of certain generated output. |
dec |
Converts integer input or generates integer output in decimal base. |
hex |
Converts integer input or generates integer output in hexadecimal base. |
oct |
Converts integer input or generates integer output in octal base. |
fixed |
Generates floating-point output in fixed-point notation. |
scientific |
Generates floating-point output in scientific notation. |
Skipws |
Skips leading white space before certain input operation. |
iostate
The type iostate is a bitmask type. Setting its elements has the following effects:
badbit |
Indicates a loss of integrity in an input or output sequence. |
eofbit |
Indicates that an input operation reached the end of an input sequence. |
failbit |
Indicates that an input operation failed to read the expected characters, or that an output operation failed to generate the desired characters. |
openmode
The type openmode is a bitmask type. Setting its elements has the following effects:
app |
Seeks to the end before writing. |
ate |
Opens and seek to end immediately after opening. |
binary |
Performs input and output in binary mode. |
in |
Opens for input. |
out |
Opens for output. |
trunc |
Truncates an existing stream when opening. |
seekdir
The type seekdir is a bitmask type. Setting its elements has the following effects:
beg |
Requests a seek relative to the beginning of the stream. |
cur |
Requests a seek relative to the current position within the sequence. |
end |
Requests a seek relative to the current end of the sequence. |
event_callback
The type event_callback is the type of the callback function used as a parameter in the function register_callback. These functions allow you to use the iword, pword mechanism in an exception-safe environment.
Public Constructor
ios_base();
The ios_base members have an indeterminate value after construction.
Public Destructor
~ios_base();
Destroys an object of class ios_base. Calls each registered callback pair (fn, index) as (*fn)(erase_event,*this, index) at such a time that any ios_base member function called from within fn has well-defined results.
Public Member Functions
ios_base& copyfmt(const ios_base& rhs);
Assigns to the member objects of *this the corresponding member objects of rhs. The content of the union pointed at by pword and iword is copied, not the pointers itself. Before copying any parts of rhs, calls each registered callback pair (fn,index) as (*fn)(erase_even,*this, index). After all parts have been replaced, calls each callback pair that was copied from rhs as (*fn)(copy_event,*this,index).
fmtflags flags() const;
Returns the format control information for both input and output
fmtflags flags(fmtflags fmtfl);
Saves the format control information, then sets it to fmtfl and returns the previously saved value.
locale getloc() const;
Returns the imbued locale, to be used to perform locale-dependent input and output operations. The default locale, locale::locale(), is used if no other locale object has been imbued in the stream by a call to the imbue function.
locale imbue(const locale& loc);
Saves the value returned by getloc() then assigns loc to a private variable and calls each registered callback pair (fn, index) as (*fn)(imbue_event,*this, index). It then returns the previously saved value.
bool is_sync();
Returns true if the C++ standard streams and the standard C streams are synchronized, otherwise returns false. This function is not part of the C++ standard.
long& iword(int idx);
Returns userwords_[idx].lword. If userwords_ is a null pointer, allocates a union of long and void* of unspecified size and stores a pointer to its first element in userwords_. The function then extends the union pointed at by userwords_ as necessary to include the element userwords_[idx]. Each newly allocated element of the union is initialized to zero. The reference returned may become invalid after another call to the object's iword or pword member with a different index, after a call to its copyfmt member, or when the object is destroyed.
streamsize precision() const;
Returns the precision (number of digits after the decimal point) to generate on certain output conversions.
streamsize precision(streamsize prec);
Saves the precision then sets it to prec and returns the previously saved value.
void*& pword(int idx);
Returns userword_[idx].pword. If userwords_ is a null pointer, allocates a union of long and void* of unspecified size and stores a pointer to its first element in userwords_. The function then extends the union pointed at by userwords_ as necessary to include the element userwords_[idx]. Each newly allocated element of the array is initialized to zero. The reference returned may become invalid after another call to the object's pword or iword member with different index, after a call to its copyfmt member, or when the object is destroyed.
void register_callback(event_callback fn, int index);
Registers the pair (fn, index) such that during calls to imbue(),copyfmt(), or ~ios_base(), the function fn is called with argument index. Functions registered are called when an event occurs, in opposite order of registration. Functions registered while a callback function is active are not called until the next event. Identical pairs are not merged; a function registered twice is called twice per event.
fmtflags setf(fmtflags fmtfl);
Saves the format control information, then sets it to fmtfl and returns the previously saved value.
fmtflags setf(fmtflags fmtfl, fmtflags mask);
Saves the format control information, then clears mask in flags(),sets fmtfl & mask in flags() and returns the previously saved value.
bool sync_with_stdio(bool sync = true);
Called with a false argument, it allows the C++ standard streams to operate independently of the standard C streams, which will greatly improve performance when using the C++ standard streams. Called with a true argument it restores the default synchronization. The return value of the function is the status of the synchronization at the time of the call.
void unsetf(fmtflags mask);
Clears mask in flags().
streamsize width() const;
Returns the field width (number of characters) to generate on certain output conversions.
streamsize width(streamsize wide);
Saves the field width then sets it to wide and returns the previously saved value.
static int xalloc();
Returns the next static index that can be used with pword and iword. This is useful if you want to share data between several stream objects.
The Class failure
The class failure defines the base class for the types of all objects thrown as exceptions, by functions in the iostreams library, to report errors detected during stream buffer operations.
explicit failure(const string& msg);
Constructs an object of class failure, initializing the base class with exception(msg).
const char* what() const;
Returns the message msg with which the exception was created.
Class Init
The class Init describes an object whose construction ensures the construction of the eight objects declared in <iostream> that associate file stream buffers with the standard C streams.
Non-Member Functions
ios_base& boolalpha(ios_base& str);
Calls str.setf(ios_base::boolalpha) and returns str.
ios_base& dec(ios_base& str);
Calls str.setf(ios_base::dec, ios_base::basefield) and returns str.
ios_base& fixed(ios_base& str);
Calls str.setf(ios_base::fixed, ios_base::floatfield) and returns str.
ios_base& hex(ios_base& str);
Calls str.setf(ios_base::hex, ios_base::basefield) and returns str.
ios_base& internal(ios_base& str);
Calls str.setf(ios_base::internal, ios_base::adjustfield) and returns str.
ios_base& left(ios_base& str);
Calls str.setf(ios_base::left, ios_base::adjustfield) and returns str.
ios_base& noboolalpha(ios_base& str);
Calls str.unsetf(ios_base::boolalpha) and returns str.
ios_base& noshowbase(ios_base& str);
Calls str.unsetf(ios_base::showbase) and returns str.
ios_base& noshowpoint(ios_base& str);
Calls str.unsetf(ios_base::showpoint) and returns str.
ios_base& noshowpos(ios_base& str);
Calls str.unsetf(ios_base::showpos) and returns str.
ios_base& noskipws(ios_base& str);
Calls str.unsetf(ios_base::skipws) and returns str.
ios_base& nounitbuf(ios_base& str);
Calls str.unsetf(ios_base::unitbuf) and returns str.
ios_base& nouppercase(ios_base& str);
Calls str.unsetf(ios_base::uppercase) and returns str.
ios_base& oct(ios_base& str);
Calls str.setf(ios_base::oct, ios_base::basefield) and returns str.
ios_base& right(ios_base& str);
Calls str.setf(ios_base::right, ios_base::adjustfield) and returns str.
ios_base& scientific(ios_base& str);
Calls str.setf(ios_base::scientific, ios_base::floatfield) and returns str.
ios_base& showbase(ios_base& str);
Calls str.setf(ios_base::showbase) and returns str.
ios_base& showpoint(ios_base& str);
Calls str.setf(ios_base::showpoint) and returns str.
ios_base& showpos(ios_base& str);
Calls str.setf(ios_base::showpos) and returns str.
ios_base& skipws(ios_base& str);
Calls str.setf(ios_base::skipws) and returns str.
ios_base& unitbuf(ios_base& str);
Calls str.setf(ios_base::unitbuf) and returns str.
ios_base& uppercase(ios_base& str);
Calls str.setf(ios_base::uppercase) and returns str.
See Also
basic_ios(3C++), basic_istream(3C++), basic_ostream(3C++), char_traits(3C++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Section 27.4.3
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
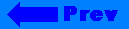
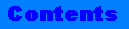
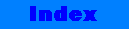
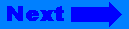
©Copyright 1996, Rogue Wave Software, Inc.