Personal tools
basic_ios

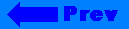
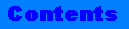
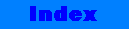
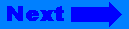
Click on the banner to return to the class reference home page.
basic_ios
basic_Iosios_base
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Public Constructors
- Public Destructor
- Public Member Functions
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | ||
char_type int_type ios ios_type |
off_type ostream_type pos_type streambuf_type |
traits_type wios |
Member Functions | |
bad() clear() copyfmt() eof() exceptions() fail() fill() good() imbue() init() |
narrow() operator!() rdbuf() rdstate() setstate() tie() void*() widen() |
Synopsis
#include <ios> template<class charT, class traits = char_traits<charT> > class basic_ios : public ios_base
Description
The class basic_ios is a base class that provides the common functionality required by all streams. It maintains state information that reflects the integrity of the stream and stream buffer. It also maintains the link between the stream classes and the stream buffers classes via the rdbuf member functions. Classes derived from basic_ios specialize operations for input, and output.
Interface
template<class charT, class traits = char_traits<charT> > class basic_ios : public ios_base { public: typedef basic_ios<charT, traits> ios_type; typedef basic_streambuf<charT, traits> streambuf_type; typedef basic_ostream<charT, traits> ostream_type; typedef typename traits::char_type char_type; typedef traits traits_type; typedef typename traits::int_type int_type; typedef typename traits::off_type off_type; typedef typename traits::pos_type pos_type; explicit basic_ios(basic_streambuf<charT, traits> *sb_arg); virtual ~basic_ios(); char_type fill() const; char_type fill(char_type ch); void exceptions(iostate except); iostate exceptions() const; void clear(iostate state = goodbit); void setstate(iostate state); iostate rdstate() const; operator void*() const; bool operator!() const; bool good() const; bool eof() const; bool fail() const; bool bad() const; ios_type& copyfmt(const ios_type& rhs); ostream_type *tie() const; ostream_type *tie(ostream_type *tie_arg); streambuf_type *rdbuf() const; streambuf_type *rdbuf( streambuf_type *sb); locale imbue(const locale& loc); char narrow(charT, char) const; charT widen(char) const; protected: basic_ios(); void init(basic_streambuf<charT, traits> *sb); };
Types
char_type
The type char_type is a synonym of type traits::char_type.
ios
The type ios is an instantiation of basic_ios on char:
typedef basic_ios<char> ios;
int_type
The type int_type is a synonym of type traits::in_type.
ios_type
The type ios_type is a synonym for basic_ios<charT, traits> .
off_type
The type off_type is a synonym of type traits::off_type.
ostream_type
The type ostream_type is a synonym for basic_ostream<charT, traits> .
pos_type
The type pos_type is a synonym of type traits::pos_type.
streambuf_type
The type streambuf_type is a synonym for basic_streambuf<charT, traits> .
traits_type
The type traits_type is a synonym for the template parameter traits.
wios
The type wios is an instantiation of basic_ios on wchar_t:
typedef basic_ios<wchar_t> wios;
Public Constructors
explicit basic_ios(basic_streambuf<charT, traits>* sb);
Constructs an object of class basic_ios, assigning initial values to its member objects by calling init(sb). If sb is a null pointer, the stream is positioned in error state, by triggering its badbit.
basic_ios();
Constructs an object of class basic_ios leaving its member objects uninitialized. The object must be initialized by calling the init member function before using it.
Public Destructor
virtual ~basic_ios();
Destroys an object of class basic_ios.
Public Member Functions
bool bad() const;
Returns true if badbit is set in rdstate().
void clear(iostate state = goodbit);
If (state & exception()) == 0, returns. Otherwise, the function throws an object of class ios_base::failure. After the call returns state == rdstate().
basic_ios& copyfmt(const basic_ios& rhs);
rdstate() and rdbuf() are left unchanged
calls ios_base::copyfmt
exceptions() is altered last by calling exceptions(rhs.exceptions())
Assigns to the member objects of *this the corresponding member objects of rhs, with the following exceptions:
bool eof() const;
Returns true if eofbit is set in rdstate().
iostate exceptions() const;
Returns a mask that determines what elements set in rdstate() cause exceptions to be thrown.
void exceptions(iostate except);
Set the exception mask to except then calls clear(rdstate()).
bool fail() const;
Returns true if failbit or badbit is set in rdstate().
char_type fill() const;
Returns the character used to pad (fill) an output conversion to the specified field width.
char_type fill(char_type fillch);
Saves the field width value then replaces it by fillch and returns the previously saved value.
bool good() const;
Returns rdstate() == 0.
locale imbue(const locale& loc);
Saves the value returned by getloc() then assigns loc to a private variable and if rdbuf() != 0 calls rdbuf()->pubimbue(loc) and returns the previously saved value.
void init(basic_streambuf<charT,traits>* sb);
Performs the following initialization:
rdbuf() sb tie() 0 rdstate() goodbit if sb is not null otherwise badbit exceptions() goodbit flags() skipws | dec width() 0 precision() 6 fill() the space character getloc() locale::locale()
char narrow(charT c, char dfault) const;
Uses the stream's locale to convert the wide character c to a tiny character, and then returns it. If no conversion exists, it returns the character dfault.
bool operator!() const;
Returns fail() ? 1 : 0;
streambuf_type* rdbuf() const;
Returns a pointer to the stream buffer associated with the stream.
streambuf_type* rdbuf(streambuf_type* sb);
Associates a stream buffer with the stream. All the input and output will be directed to this stream buffer. If sb is a null pointer, the stream is positioned in error state, by triggering its badbit.
iostate rdstate() const;
Returns the control state of the stream.
void setstate(iostate state);
Calls clear(rdstate() | state).
ostream_type* tie() const;
Returns an output sequence that is tied to (synchronized with) the sequence controlled by the stream buffer.
ostream_type* tie(ostream_type* tiestr);
Saves the tie() value then replaces it by tiestr and returns the value previously saved.
operator void*() const;
Returns fail() ? 0 : 1;
charT widen(char c) const;
Uses the stream's locale to convert the tiny character c to a wide character, then returns it.
See Also
ios_base(3C++), basic_istream(3C++), basic_ostream(3C++), basic_streambuf(3C++), char_traits(3C++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++.
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
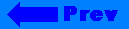
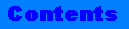
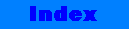
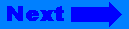
©Copyright 1996, Rogue Wave Software, Inc.