Personal tools
basic_stringbuf

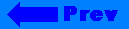
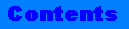
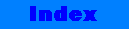
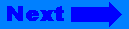
Click on the banner to return to the class reference home page.
basic_stringbuf
basic_stringbufbasic_streambuf
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors
- Destructor
- Member Functions
- Examples
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Member Functions |
overflow() pbackfail() seekoff() seekpos() setbuf() str() underflow() xsputn() |
Synopsis
#include <sstream> template<class charT, class traits = char_traits<charT>, class Allocator = allocator<void> > class basic_stringbuf : public basic_streambuf<charT, traits>
Description
The class basic_stringbuf is derived from basic_streambuf. Its purpose is to associate the input or output sequence with a sequence of arbitrary characters. The sequence can be initialized from, or made available as, an object of class basic_string. Each object of type basic_stringbuf<charT, traits,Allocator> controls two character sequences:
A character input sequence;
A character output sequence.
Note: see basic_streambuf.
The two sequences are related to each other, but are manipulated separately. This allows you to read and write characters at different positions in objects of type basic_stringbuf without any conflict (as opposed to the basic_filebuf objects, in which a joint file position is maintained).
Interface
template<class charT, class traits = char_traits<charT>, class allocator<void> > class basic_stringbuf : public basic_streambuf<charT, traits> { public: typedef basic_ios<charT, traits> ios_type; typedef basic_string<charT, traits, Allocator> string_type; typedef charT char_type; typedef typename traits::int_type int_type; typedef typename traits::pos_type pos_type; typedef typename traits::off_type off_type; explicit basic_stringbuf(ios_base::openmode which = (ios_base::in | ios_base::out)); explicit basic_stringbuf(const string_type& str, ios_base::openmode which = (ios_base::in | ios_base::out)); virtual ~basic_stringbuf(); string_type str() const; void str(const string_type& str_arg); protected: virtual int_type overflow(int_type c = traits::eof()); virtual int_type pbackfail(int_type c = traits::eof()); virtual int_type underflow(); virtual basic_streambuf<charT,traits>* setbuf(char_type *s,streamsize n); virtual pos_type seekoff(off_type off, ios_base::seekdir way, ios_base::openmode which = ios_base::in | ios_base::out); virtual pos_type seekpos(pos_type sp, ios_base::openmode which = ios_base::in | ios_base::out); virtual streamsize xsputn(const char_type* s, streamsize n); };
Types
char_type
The type char_type is a synonym for the template parameter charT.
ios_type
The type ios_type is an instantiation of class basic_ios on type charT.
off_type
The type off_type is a synonym of type traits::off_type.
pos_type
The type pos_type is a synonym of type traits::pos_type.
string_type
The type string_type is an instantiation of class basic_string on type charT.
stringbuf
The type stringbuf is an instantiation of class basic_stringbuf on type char:
typedef basic_stringbuf<char> stringbuf;
traits_type
The type traits_type is a synonym for the template parameter traits.
wstringbuf
The type wstringbuf is an instantiation of class basic_stringbuf on type wchar_t:
typedef basic_stringbuf<wchar_t> wstringbuf;
Constructors
explicit basic_stringbuf(ios_base::openmode which = ios_base::in | ios_base::out);
Constructs an object of class basic_stringbuf, initializing the base class with basic_streambuf(), and initializing the open mode with which.
explicit basic_stringbuf(const string_type& str, ios_base::openmode which = ios_base::in | ios_base::out);
Constructs an object of class basic_stringbuf, initializing the base class with basic_streambuf(), and initializing the open mode with which. The string object str is copied to the underlying buffer. If the opening mode is in, initialize the input sequence to point at the first character of the buffer. If the opening mode is out, it initializes the output sequence to point at the first character of the buffer. If the opening mode is out | app, it initializes the output sequence to point at the last character of the buffer.
Destructor
virtual ~basic_stringbuf();
Destroys an object of class basic_stringbuf.
Member Functions
int_type overflow(int_type c = traits::eof() );
If the output sequence has a put position available, and c is not traits::eof(), then this functions writes c into it. If there is no position available, the function increases the size of the buffer by allocating more memory and then writes c at the new current put position. If the operation fails, the function returns traits::eof(). Otherwise it returns traits::not_eof(c).
int_type pbackfail( int_type c = traits::eof() );
Puts back the character designated by c into the input sequence. If traits::eq_int_type(c,traits::eof()) returns true, the function moves the input sequence one position backward. If the operation fails, the function returns traits::eof(). Otherwise it returns traits::not_eof(c).
pos_type seekoff(off_type off, ios_base::seekdir way, ios_base::openmode which = ios_base::in | ios_base::out);
If the open mode is in | out, this function alters the stream position of both the input and the output sequences. If the open mode is in, it alters the stream position of only the input sequence, and if it is out, it alters the stream position of the output sequence. The new position is calculated by combining the two parameters off (displacement) and way (reference point). If the current position of the sequence is invalid before repositioning, the operation fails and the return value is pos_type(off_type(-1)). Otherwise the function returns the current new position.
pos_type seekpos(pos_type sp,ios_base::openmode which = ios_base::in | ios_base::out);
If the open mode is in | out, seekpos() alters the stream position of both the input and the output sequences. If the open mode is in, it alters the stream position of the input sequence only, and if the open mode is out, it alters the stream position of the output sequence only. If the current position of the sequence is invalid before repositioning, the operation fails and the return value is pos_type(off_type(-1)). Otherwise the function returns the current new position.
basic_streambuf<charT,traits>* setbuf(char_type*s, streamsize n);
If the string buffer object is opened in output mode, proceed as follows:
if s is not a null pointer and n is greater than the content of the current buffer, replace it (copy its contents) by the buffer of size n pointed at by s. In the case where s is a null pointer and n is greater than the content of the current buffer, resize it to size n. If the function fails, it returns a null pointer.
string_type str() const;
Returns a string object of type string_type whose content is a copy of the underlying buffer contents.
void str(const string_type& str_arg);
Clears the underlying buffer and copies the string object str_arg into it. If the opening mode is in, initializes the input sequence to point at the first character of the buffer. If the opening mode is out, the function initializes the output sequence to point at the first character of the buffer. If the opening mode is out | app, it initializes the output sequence to point at the last character of the buffer.
int_type underflow();
If the input sequence has a read position available, the function returns the contents of this position. Otherwise it tries to expand the input sequence to match the output sequence and if possible returns the content of the new current position. The function returns traits::eof() to indicate failure.
streamsize xsputn(const char_type* s, streamsize n);
Writes up to n characters to the output sequence. The characters written are obtained from successive elements of the array whose first element is designated by s. The function returns the number of characters written.
Examples
// stdlib/examples/manual/stringbuf.cpp // #include<iostream> #include<sstream> #include<string> void main ( ) { using namespace std; // create a read/write string-stream object on tiny char // and attach it to an ostringstream object ostringstream out_1(ios_base::in | ios_base::out); // tie the istream object to the ostringstream object istream in_1(out_1.rdbuf()); // output to out_1 out_1 << "Here is the first output"; // create a string object on tiny char string string_ex("l'heure est grave !"); // open a read only string-stream object on tiny char // and initialize it istringstream in_2(string_ex); // output in_1 to the standard output cout << in_1.str() << endl; // output in_2 to the standard output cout << in_2.rdbuf() << endl; // reposition in_2 at the beginning in_2.seekg(0); stringbuf::pos_type pos; // get the current put position // equivalent to // out_1.tellp(); pos = out_1.rdbuf()->pubseekoff(0,ios_base::cur, ios_base::out); // append the content of stringbuffer // pointed at by in_2 to the one // pointed at by out_1 out_1 << ' ' << in_2.rdbuf(); // output in_1 to the standard output cout << in_1.str() << endl; // position the get sequence // equivalent to // in_1.seekg(pos); in_1.rdbuf()->pubseekpos(pos, ios_base::in); // output "l'heure est grave !" cout << in_1.rdbuf() << endl << endl; }
See Also
char_traits(3C++), ios_base(3C++), basic_ios(3C++), basic_streambuf(3C++), basic_string(3C++), basic_istringstream(3C++), basic_ostringstream(3C++), basic_stringstream(3C++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Section 27.7.1
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
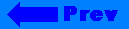
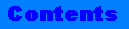
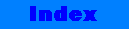
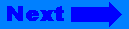
©Copyright 1996, Rogue Wave Software, Inc.