Personal tools
basic_filebuf

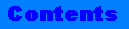
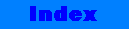
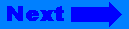
Click on the banner to return to the class reference home page.
basic_filebuf
basic_filebufbasic_streambuf
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors
- Destructor
- Member Functions
- Examples
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Member Functions | |
close() is_open() open() overflow() pbackfail() seekoff() seekpos() setbuf() sync() underflow() |
xsputn() |
Synopsis
#include <fstream> template<class charT, class traits = char_traits<charT> > class basic_filebuf : public basic_streambuf<charT, traits>
Description
The template class basic_filebuf is derived from basic_streambuf. It associates the input or output sequence with a file. Each object of type basic_filebuf<charT, traits> controls two character sequences:
a character input sequence
a character output sequence
The restrictions on reading and writing a sequence controlled by an object of class basic_filebuf<charT,traits> are the same as for reading and writing with the Standard C library files.
If the file is not open for reading the input sequence cannot be read. If the file is not open for writing the output sequence cannot be written. A joint file position is maintained for both the input and output sequences.
A file provides byte sequences. So the basic_filebuf class treats a file as the external source (or sink) byte sequence. In order to provide the contents of a file as wide character sequences, a wide-oriented file buffer called wfilebuf converts wide character sequences to multibytes character sequences (and vice versa) according to the current locale being used in the stream buffer.
Interface
template<class charT, class traits = char_traits<charT> > class basic_filebuf : public basic_streambuf<charT, traits> { public: typedef traits traits_type; typedef charT char_type; typedef typename traits::int_type int_type; typedef typename traits::pos_type pos_type; typedef typename traits::off_type off_type; basic_filebuf(); basic_filebuf(int fd); virtual ~basic_filebuf(); bool is_open() const; basic_filebuf<charT, traits>* open(const char *s, ios_base::openmode, long protection = 0666); basic_filebuf<charT, traits>* open(int fd); basic_filebuf<charT, traits>* close(); protected: virtual int showmanyc(); virtual int_type overflow(int_type c = traits::eof()); virtual int_type pbackfail(int_type c = traits::eof()); virtual int_type underflow(); virtual basic_streambuf<charT,traits>* setbuf(char_type *s,streamsize n); virtual pos_type seekoff(off_type off, ios_base::seekdir way, ios_base::openmode which = ios_base::in | ios_base::out); virtual pos_type seekpos(pos_type sp, ios_base::openmode which = ios_base::in | ios_base::out); virtual int sync(); virtual streamsize xsputn(const char_type* s, streamsize n); };
Types
char_type
The type char_type is a synonym for the template parameter charT.
filebuf
The type filebuf is an instantiation of class basic_filebuf on type char:
typedef basic_filebuf<char> filebuf;
int_type
The type int_type is a synonym of type traits::in_type.
off_type
The type off_type is a synonym of type traits::off_type.
pos_type
The type pos_type is a synonym of type traits::pos_type.
traits_type
The type traits_type is a synonym for the template parameter traits.
wfilebuf
The type wfilebuf is an instantiation of class basic_filebuf on type wchar_t:
typedef basic_filebuf<wchar_t> wfilebuf;
Constructors
basic_filebuf();
Constructs an object of class basic_filebuf<charT,traits>, initializing the base class with basic_streambuf<charT,traits>().
basic_filebuf(int fd);
Constructs an object of class basic_filebuf<charT,traits>, initializing the base class with basic_streambuf<charT,traits>(), then calls open(fd). This function is not described in the C++ standard, and is provided as an extension in order to manipulate pipes, sockets or other UNIX devices, that can be accessed through file descriptors.
Destructor
virtual ~basic_filebuf();
Calls close() and destroys the object.
Member Functions
basic_filebuf<charT,traits>* close();
If is_open() == false, returns a null pointer. Otherwise, closes the file, and returns *this.
bool is_open() const;
Returns true if the associated file is open.
basic_filebuf<charT,traits>* open(const char* s, ios_base::openmode mode, long protection = 0666);
If is_open() == true, returns a null pointer. Otherwise opens the file, whose name is stored in the null-terminated byte-string s. The file open modes are given by their C-equivalent description (see the C function fopen):
in "w" in|binary "rb" out "w" out|app "a" out|binary "wb" out|binary|app "ab" out|in "r+" out|in|app "a+" out|in|binary "r+b" out|in|binary|app "a+b" trunc|out "w" trunc|out|binary "wb" trunc|out|in "w+" trunc|out|in|binary "w+b"
The third argument, protection, is used as the file permission. It does not appear in the Standard C++ description of the function open and is provided as an extension. It determines the file read/write/execute permissions under UNIX. It is more limited under DOS since files are always readable and do not have special execute permission. If the open function fails, it returns a null pointer.
basic_filebuf<charT,traits>* open(int fd);
Attaches the file previously opened and identified by its file descriptor fd, to the basic_filebuf object. This function is not described in the C++ standard, and is provided as an extension in order to manipulate pipes, sockets or other UNIX devices, that can be accessed through file descriptors.
int_type overflow(int_type c = traits::eof() );
If the output sequence has a put position available, and c is not traits::eof(), then write c into it. If there is no position available, the function output the content of the buffer to the associated file and then write c at the new current put position. If the operation fails, the function returns traits::eof(). Otherwise it returns traits::not_eof(c). In wide characters file buffer, overflow converts the internal wide characters to their external multibytes representation by using the locale::codecvt facet located in the locale object imbued in the stream buffer.
int_type pbackfail(int_type c = traits::eof() );
Puts back the character designated by c into the input sequence. If traits::eq_int_type(c,traits::eof()) returns true, move the input sequence one position backward. If the operation fails, the function returns traits::eof(). Otherwise it returns traits::not_eof(c).
pos_type seekoff(off_type off, ios_base::seekdir way, ios_base::openmode which = ios_base::in | ios_base::out);
If the open mode is in | out, alters the stream position of both the input and the output sequence. If the open mode is in, alters the stream position of only the input sequence, and if it is out, alters the stream position of only the output sequence. The new position is calculated by combining the two parameters off (displacement) and way (reference point). If the current position of the sequence is invalid before repositioning, the operation fails and the return value is pos_type(off_type(-1)). Otherwise the function returns the current new position. File buffers using locale::codecvt facet performing state dependent conversion, only support seeking to the beginning of the file, to the current position, or to a position previously obtained by a call to one of the iostreams seeking functions.
pos_type seekpos(pos_type sp,ios_base::openmode which = ios_base::in | ios_base::out);
If the open mode is in | out, alters the stream position of both the input and the output sequence. If the open mode is in, alters the stream position of only the input sequence, and if it is out, alters the stream position of only the output sequence. If the current position of the sequence is invalid before repositioning, the operation fails and the return value is pos_type(off_type(-1)). Otherwise the function returns the current new position. File buffers using locale::codecvt facet performing state dependent conversion, only support seeking to the beginning of the file, to the current position, or to a position previously obtained by a call to one of the iostreams seeking functions.
basic_filebuf<charT,traits>* setbuf(char_type*s, streamsize n);
If s is not a null pointer, output the content of the current buffer to the associated file, then delete the current buffer and replace it by s. Otherwise resize the current buffer to size n after outputting its content to the associated file if necessary.
int sync();
Synchronizes the content of the external file, with its image maintained in memory by the file buffer. If the function fails, it returns -1, otherwise it returns 0.
int_type underflow();
If the input sequence has a read position available, returns the content of this position. Otherwise fills up the buffer by reading characters from the associated file and if it succeeds, returns the content of the new current position. The function returns traits::eof() to indicate failure. In wide characters file buffer, underflow converts the external multibytes characters to their wide character representation by using the locale::codecvt facet located in the locale object imbued in the stream buffer.
streamsize xsputn(const char_type* s, streamsize n);
Writes up to n characters to the output sequence. The characters written are obtained from successive elements of the array whose first element is designated by s. The function returns the number of characters written.
Examples
// // stdlib/examples/manual/filebuf.cpp // #include<iostream> #include<fstream> void main ( ) { using namespace std; // create a read/write file-stream object on tiny char // and attach it to the file "filebuf.out" ofstream out("filebuf.out",ios_base::in | ios_base::out); // tie the istream object to the ofstream object istream in(out.rdbuf()); // output to out out << "Il errait comme un ame en peine"; // seek to the beginning of the file in.seekg(0); // output in to the standard output cout << in.rdbuf() << endl; // close the file "filebuf.out" out.close(); // open the existing file "filebuf.out" // and truncate it out.open("filebuf.out",ios_base::in | ios_base::out | ios_base::trunc); // set the buffer size out.rdbuf()->pubsetbuf(0,4096); // open the source code file ifstream ins("filebuf.cpp"); //output it to filebuf.out out << ins.rdbuf(); // seek to the beginning of the file out.seekp(0); // output the all file to the standard output cout << out.rdbuf(); }
See Also
char_traits(3C++), ios_base(3C++), basic_ios(3C++), basic_streambuf(3C++), basic_ifstream(3C++), basic_ofstream(3C++), basic_fstream(3C++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Section 27.8.1.1
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
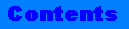
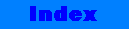
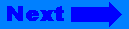
©Copyright 1996, Rogue Wave Software, Inc.