Personal tools
strstreambuf

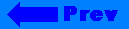
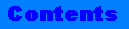
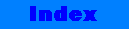
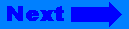
Click on the banner to return to the class reference home page.
strstreambuf
strstreambufbasic_streambuf
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors
- Destructors
- Member Functions
- Examples
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Member Functions | |
freeze() overflow() pbackfail() pcount() seekoff() seekpos() setbuf() str() underflow() xsputn() |
Synopsis
#include <strstream> class strstreambuf : public basic_streambuf<char>
Description
The class strstreambuf is derived from basic_streambuf specialized on type char to associate possibly the input sequence and possibly the output sequence with a tiny character array, whose elements store arbitrary values.
Each object of type strstreambuf controls two character sequences:
A character input sequence;
A character output sequence.
Note: see basic_streambuf.
The two sequences are related to each other, but are manipulated separately. This means that you can read and write characters at different positions in objects of type strstreambuf without any conflict (in opposition to the basic_filebuf objects).
The underlying array has several attributes:
allocated, set when a dynamic array has been allocated, and hence should be freed by the destructor of the strstreambuf object.
constant, set when the array has const elements, so the output sequence cannot be written.
dynamic, set when the array object is allocated (or reallocated) as necessary to hold a character sequence that can change in length.
frozen, set when the program has requested that the array will not be altered, reallocated, or freed.
Interface
class strstreambuf : public basic_streambuf<char> { public: typedef char_traits<char> traits; typedef basic_ios<char, traits> ios_type; typedef char char_type; typedef typename traits::int_type int_type; typedef typename traits::pos_type pos_type; typedef typename traits::off_type off_type; explicit strstreambuf(streamsize alsize = 0); strstreambuf(void *(*palloc)(size_t), void (*pfree)(void *)); strstreambuf(char *gnext, streamsize n, char *pbeg = 0); strstreambuf(unsigned char *gnext, streamsize n, unsigned char *pbeg = 0); strstreambuf(signed char *gnext, streamsize n, signed char *pbeg = 0); strstreambuf(const char *gnext, streamsize n); strstreambuf(const unsigned char *gnext, streamsize n); strstreambuf(const signed char *gnext, streamsize n); virtual ~strstreambuf(); void freeze(bool f = 1); char *str(); int pcount() const; protected: virtual int_type overflow(int_type c = traits::eof()); virtual int_type pbackfail(int_type c = traits::eof()); virtual int_type underflow(); virtual pos_type seekoff(off_type, ios_type::seekdir way, ios_type::openmode which = ios_type::in | ios_type::out); virtual pos_type seekpos(pos_type sp, ios_type::openmode which = ios_type::in | ios_type::out); virtual streambuf* setbuf(char *s, streamsize n); virtual streamsize xsputn(const char_type* s, streamsize n); };
Types
char_type
The type char_type is a synonym of type char.
int_type
The type int_type is a synonym of type traits::in_type.
ios_type
The type ios_type is an instantiation of class basic_ios on type char.
off_type
The type off_type is a synonym of type traits::off_type.
pos_type
The type pos_type is a synonym of type traits::pos_type.
traits
The type traits is a synonym of type char_traits<char>.
Constructors
explicit strstreambuf(streamsize alsize = 0);
Constructs an object of class strstreambuf, initializing the base class with streambuf(). After initialization the strstreambuf object is in dynamic mode and its array object has a size of alsize.
strstreambuf(void* (*palloc)(size_t), void (*pfree)(void*));
Constructs an object of class strstreambuf, initializing the base class with streambuf(). After initialization the strstreambuf object is in dynamic mode. The function used to allocate memory is pointed at by void* (*palloc)(size_t) and the one used to free memory is pointed at by void (*pfree)(void*).
strstreambuf(char* gnext, streamsize n, char* pbeg = 0); strstreambuf(signed char* gnext, streamsize n, signed char* pbeg = 0); strstreambuf(unsigned char* gnext, streamsize n, unsigned char* pbeg = 0);
Constructs an object of class strstreambuf, initializing the base class with streambuf(). The argument gnext point to the first element of an array object whose number of elements is:
n, if n > 0 ::strlen(gnext), if n == 0 INT_MAX, if n < 0
If pbeg is a null pointer set only the input sequence to gnext, otherwise set also the output sequence to pbeg.
strstreambuf(const char* gnext, streamsize n); strstreambuf(const signed char* gnext, streamsize n); strstreambuf(const unsigned char* gnext, streamsize n);
Constructs an object of class strstreambuf, initializing the base class with streambuf(). The argument gnext point to the first element of an array object whose number of elements is:
n, if n > 0 ::strlen(gnext), if n == 0 INT_MAX, if n < 0
Set the input sequence to gnext and the mode to constant.
Destructors
virtual ~strstreambuf();
Destroys an object of class strstreambuf. The function frees the dynamically allocated array object only if allocated is set and frozen is not set.
Member Functions
void freeze(bool freezefl = 1);
If freezefl is false, the function sets the freeze status to frozen.
Otherwise, it clears the freeze status.
If the mode is dynamic, alters the freeze status of the dynamic array as follows:
int_type overflow( int_type c = traits::eof() );
If the output sequence has a put position available, and c is not traits::eof(), then write c into it. If there is no position available, the function grow the size of the array object by allocating more memory and then write c at the new current put position. If dynamic is not set or if frozen is set the operation fails. The function returns traits::not_eof(c), except if it fails, in which case it returns traits::eof().
int_type pbackfail( int_type c = traits::eof() );
Puts back the character designated by c into the input sequence. If traits::eq_int_type(c,traits::eof()) returns true, move the input sequence one position backward. If the operation fails, the function returns traits::eof(). Otherwise it returns traits::not_eof(c).
int pcount() const;
Returns the size of the output sequence.
pos_type seekoff(off_type off, ios_base::seekdir way, ios_base::openmode which = ios_base::in | ios_base::out);
If the open mode is in | out, alters the stream position of both the input and the output sequence. If the open mode is in, alters the stream position of only the input sequence, and if it is out, alters the stream position of only the output sequence. The new position is calculated by combining the two parameters off (displacement) and way (reference point). If the current position of the sequence is invalid before repositioning, the operation fails and the return value is pos_type(off_type(-1)). Otherwise the function returns the current new position.
pos_type seekpos(pos_type sp,ios_base::openmode which = ios_base::in | ios_base::out);
If the open mode is in | out, alters the stream position of both the input and the output sequence. If the open mode is in, alters the stream position of only the input sequence, and if it is out, alters the stream position of only the output sequence. If the current position of the sequence is invalid before repositioning, the operation fails and the return value is pos_type(off_type(-1)). Otherwise the function returns the current new position.
strstreambuf* setbuf(char* s, streamsize n);
If dynamic is set and freeze is not, proceed as follows:
If s is not a null pointer and n is greater than the number of characters already in the current array, replaces it (copy its contents) by the array of size n pointed at by s.
char* str();
Calls freeze(), then returns the beginning pointer for the input sequence.
int_type underflow();
If the input sequence has a read position available, returns the content of this position. Otherwise tries to expand the input sequence to match the output sequence and if possible returns the content of the new current position. The function returns traits::eof() to indicate failure.
In the case where s is a null pointer and n is greater than the number of characters already in the current array, resize it to size n.
If the function fails, it returns a null pointer.
streamsize xsputn(const char_type* s, streamsize n);
Writes up to n characters to the output sequence. The characters written are obtained from successive elements of the array whose first element is designated by s. The function returns the number of characters written.
Examples
// // stdlib/examples/manual/strstreambuf.cpp // #include<iostream> #include<strstream> #include<iomanip> void main ( ) { using namespace std; // create a read/write strstream object // and attach it to an ostrstream object ostrstream out; // tie the istream object to the ostrstream object istream in(out.rdbuf()); // output to out out << "anticonstitutionellement is a big word !!!"; // create a NTBS char *p ="Le rat des villes et le rat des champs"; // output the NTBS out << p << endl; // resize the buffer if ( out.rdbuf()->pubsetbuf(0,5000) ) cout << endl << "Success in allocating the buffer" << endl; // output the all buffer to stdout cout << in.rdbuf( ); // output the decimal conversion of 100 in hex // with right padding and a width field of 200 out << dec << setfill('!') << setw(200) << 0x100 << endl; // output the content of the input sequence to stdout cout << in.rdbuf( ) << endl; // number of elements in the output sequence cout << out.rdbuf()->pcount() << endl; // resize the buffer to a minimum size if ( out.rdbuf()->pubsetbuf(0,out.rdbuf()->pcount()) ) cout << endl << "Success in resizing the buffer" << endl; // output the content of the all array object cout << out.rdbuf()->str() << endl; }
See Also
char_traits(3C++), ios_base(3C++), basic_ios(3C++), basic_streambuf(3C++), istrstream(3c++), ostrstream(3C++), strstream(3c++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Annex D Compatibility features Section D.5
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
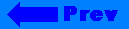
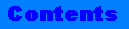
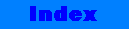
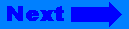
©Copyright 1996, Rogue Wave Software, Inc.