Personal tools
smanip, smanip_fill

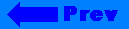
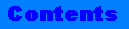
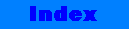
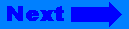
Click on the banner to return to the class reference home page.
smanip, smanip_fill
- Data Type and Member Function Indexes
- Synopsis
- Description
- interface
- Class smanip Constructor
- Class smanip_fill Constructor
- Manipulators
- Extractors
- Insertors
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Member Functions |
operator<<() >()">operator>>() resetiosflag() setbase() setfill() setiosflag() setprecision() setw() |
Synopsis
#include <iomanip> template<class T> class smanip; template<class T, class traits> class smanip_fill;
Description
The template classes smanip and smanip_fill are helper classes used to implement parameterized manipulators. The class smanip is used as the return type for manipulators that do not need to carry information about the character type of the stream they are applied to. This is the case for resetiosflags, setiosflags, setbase, setprecision and setw. The class smanip_fill is used as the return type for manipulators that do need to carry information about the character type of the stream they are applied to. This is the case for setfill.
interface
template<class T> class smanip { public: smanip(ios_base& (*pf) (ios_base&, T), T manarg); }; template<class T, class traits> class smanip_fill { public: smanip_fill(basic_ios<T, traits>& (*pf) (basic_ios<T, traits>&, T), T manarg); }; // parameterized manipulators smanip<ios_base::fmtflags> resetiosflag(ios_base::fmtflags mask); smanip<ios_base::fmtflags> setiosflag(ios_base::fmtflags mask); smanip<int> setbase(int base); smanip<int> setprecision(int n); smanip<int> setw(int n); template <class charT> smanip_fill<charT, char_traits<charT> > setfill(charT c); // overloaded extractors template <class charT, class traits, class T> basic_istream<charT,traits>& operator>>(basic_istream<charT,traits>& is, const smanip<T>& a); template <class charT, class traits> basic_istream<charT,traits>& operator>>(basic_istream<charT,traits>& is, const smanip_fill<charT,char_traits<charT> >& a); // overloaded insertors template <class charT, class traits, class T> basic_ostream<charT,traits>& operator<<(basic_ostream<charT,traits>& is, const smanip<T>& a); template <class charT, class traits> basic_ostream<charT,traits>& operator>>(basic_ostream<charT,traits>& is, const smanip_fill<charT,char_traits<charT> >& a);
Class smanip Constructor
smanip(ios_base& (*pf) (ios_base&, T), T manarg);
Constructs an object of class smanip that stores a function pointer pf, that will be called with argument manarg, in order to perform the manipulator task. The call to pf is performed in the insertor or extractor overloaded on type smanip.
Class smanip_fill Constructor
smanip_fill(basic_ios<T, traits>& (*pf) (basic_ios<T, traits>&, T), T manarg);
Constructs an object of class smanip_fill that stores a function pointer pf, that will be called with argument manarg, in order to perform the manipulator task. The call to pf is performed in the insertor or extractor overloaded on type smanip_fill.
Manipulators
smanip<ios_base::fmtflags> resetiosflag(ios_base::fmtflags mask);
Resets the ios_base::fmtflags designated by mask in the stream to which it is applied.
smanip<int> setbase(int base);
Sets the base for the output or input of integer values in the stream to which it is applied. The valid values for mask are 8, 10, 16.
template <class charT> smanip_fill<charT, char_traits<charT> > setfill(charT c);
Sets the fill character in the stream it is applied to.
smanip<ios_base::fmtflags> setiosflag(ios_base::fmtflags mask);
Sets the ios_base::fmtflags designated by mask in the stream it is applied to.
smanip<int> setprecision(int n);
Sets the precision for the output of floating point values in the stream to which it is applied.
smanip<int> setw(int n);
Set the field width in the stream to which it is applied.
Extractors
template <class charT, class traits, class T> >()">basic_istream<charT,traits>& operator>>(basic_istream<charT,traits>& is, const smanip<T>& a);
Applies the function stored in the parameter of type smanip<T>, on the stream is.
template <class charT, class traits> basic_istream<charT,traits>& operator>>(basic_istream<charT,traits>& is, const smanip_fill<charT,char_traits<charT> >& a);
Applies the function stored in the parameter of type smanip_fill<charT, char_traits<charT> >, on the stream is.
Insertors
template <class charT, class traits, class T> basic_ostream<charT,traits>& operator<<(basic_ostream<charT,traits>& os, const smanip<T>& a);
Applies the function stored in the parameter of type smanip<T>, on the stream os.
template <class charT, class traits> basic_ostream<charT,traits>& operator<<(basic_ostream<charT,traits>& os, const smanip_fill<charT,char_traits<charT> >& a);
Applies the function stored in the parameter of type smanip_fill<charT, char_traits<charT> >, on the stream os.
See Also
ios_base(3C++), basic_ios(3C++), basic_istream(3C++), basic_ostream(3C++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Section 27.6.3
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
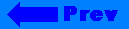
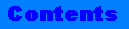
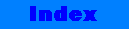
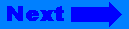
©Copyright 1996, Rogue Wave Software, Inc.