Personal tools
find

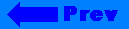
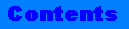
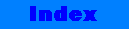
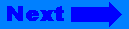
Click on the banner to return to the class reference home page.
find
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warning
- See Also
Summary
Find an occurrence of value in a sequence
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class InputIterator, class T> InputIterator find(InputIterator first, InputIterator last, const T& value);
Description
The find algorithm lets you search for the first occurrence of a particular value in a sequence. find returns the first iterator i in the range [first, last) for which the following condition holds:
*i == value.
If find does not find a match for value, it returns the iterator last.
Complexity
find performs at most last-first comparisons.
Example
// // find.cpp // #include <vector> #include <algorithm> int main() { typedef vector<int>::iterator iterator; int d1[10] = {0,1,2,2,3,4,2,2,6,7}; // Set up a vector vector<int> v1(d1,d1 + 10); // Try find iterator it1 = find(v1.begin(),v1.end(),3); // it1 = v1.begin() + 4; // Try find_if iterator it2 = find_if(v1.begin(),v1.end(),bind1st(equal_to<int>(),3)); // it2 = v1.begin() + 4 // Try both adjacent_find variants iterator it3 = adjacent_find(v1.begin(),v1.end()); // it3 = v1.begin() +2 iterator it4 = adjacent_find(v1.begin(),v1.end(),equal_to<int>()); // v4 = v1.begin() + 2 // Output results cout << *it1 << " " << *it2 << " " << *it3 << " " << *it4 << endl; return 0; } Output : 3 3 2 2
Warning
If your compiler does not support default template parameters then you need to always supply the Allocator template argument. For instance you'll have to write:
vector<int,allocator<int> >
instead of:
vector<int>
See Also
adjacent_find, find_first_of, find_if
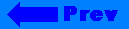
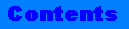
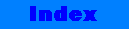
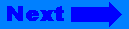
©Copyright 1996, Rogue Wave Software, Inc.