Personal tools
fill, fill_n

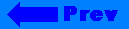
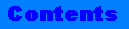
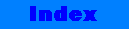
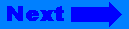
Click on the banner to return to the class reference home page.
fill, fill_n
Algorithm
Summary
Initializes a range with a given value.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class ForwardIterator, class T> void fill(ForwardIterator first, ForwardIterator last, const T& value); template <class OutputIterator, class Size, class T> void fill_n(OutputIterator first, Size n, const T& value);
Description
The fill and fill_n algorithms are used to assign a value to the elements in a sequence. fill assigns the value to all the elements designated by iterators in the range [first, last).
The fill_n algorithm assigns the value to all the elements designated by iterators in the range [first, first + n). fill_n assumes that there are at least n elements following first, unless first is an insert iterator.
Complexity
fill makes exactly last - first assignments, and fill_n makes exactly n assignments.
Example
// // fill.cpp // #include <algorithm> #include <vector> #include <iostream.h> int main() { int d1[4] = {1,2,3,4}; // // Set up two vectors // vector<int> v1(d1,d1 + 4), v2(d1,d1 + 4); // // Set up one empty vector // vector<int> v3; // // Fill all of v1 with 9 // fill(v1.begin(),v1.end(),9); // // Fill first 3 of v2 with 7 // fill_n(v2.begin(),3,7); // // Use insert iterator to fill v3 with 5 11's // fill_n(back_inserter(v3),5,11); // // Copy all three to cout // ostream_iterator<int,char> out(cout," "); copy(v1.begin(),v1.end(),out); cout << endl; copy(v2.begin(),v2.end(),out); cout << endl; copy(v3.begin(),v3.end(),out); cout << endl; // // Fill cout with 3 5's // fill_n(ostream_iterator<int,char>(cout," "),3,5); cout << endl; return 0; } Output : 9 9 9 9 7 7 7 4 11 11 11 11 11 5 5 5
Warnings
If your compiler does not support default template parameters then you need to always supply the Allocator template argument. For instance you'll have to write:
vector<int,allocator<int> >
instead of:
vector<int>
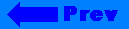
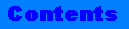
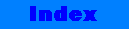
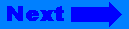
©Copyright 1996, Rogue Wave Software, Inc.