Personal tools
exception

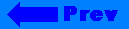
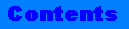
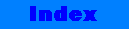
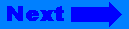
Click on the banner to return to the class reference home page.
exception
Standard Exception
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Constructors
- Destructor
- Operators
- Member Function
- Constructors for Derived Classes
- Example
Summary
Classes supporting logic and runtime errors.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Member Functions |
operator=() what() |
Synopsis
#include <exception> class exception;
Description
The class exception defines the base class for the types of objects thrown as exceptions by Standard C++ Library components, and certain expressions, to report errors detected during program execution. Users can also use these exceptions to report errors in their own programs.
Interface
class exception { public: exception () throw(); exception (const exception&) throw(); exception& operator= (const exception&) throw(); virtual ~exception () throw(); virtual const char* what () const throw(); }; class logic_error : public exception { public: logic_error (const string& what_arg); }; class domain_error : public logic_error { public: domain_error (const string& what_arg); }; class invalid_argument : public logic_error { public: invalid_argument (const string& what_arg); }; class length_error : public logic_error { public: length_error (const string& what_arg); }; class out_of_range : public logic_error { public: out_of_range (const string& what_arg); }; class runtime_error : public exception { public: runtime_error (const string& what_arg); }; class range_error : public runtime_error { public: range_error (const string& what_arg); }; class overflow_error : public runtime_error { public: overflow_error (const string& what_arg); }; class underflow_error : public runtime_error { public: underflow_error (const string& what_arg); };
Constructors
exception() throws();
Constructs an object of class exception.
exception(const exception&) throws();
The copy constructor. Copies an exception object.
Destructor
virtual ~exception() throws();
Destroys an object of class exception.
Operators
exception& operator=(const exception&) throws();
The assignment operator. Copies an exception object.
Member Function
virtual const char* what()const throws();
Returns an implementation-defined, null-terminated byte string representing a human-readable message describing the exception. The message may be a null-terminated multibyte string, suitable for conversion and display as a wstring.
Constructors for Derived Classes
logic_error::logic_error(const string& what_arg);
Constructs an object of class logic_error.
domain_error::domain_error(const string& what_arg);
Constructs an object of class domain_error.
invalid_argument::invalid_argument(const string& what_arg);
Constructs an object of class invalid_argument.
length_error::length_error(const string& what_arg);
Constructs an object of class length_error.
out_of_range::out_of_range(const string& what_arg);
Constructs an object of class out_of_range.
runtime_error::runtime_error(const string& what_arg);
Constructs an object of class runtime_error.
range_error::range_error(const string& what_arg);
Constructs an object of class range_error.
overflow_error::overflow_error(const string& what_arg);
Constructs an object of class overflow_error.
underflow_error::underflow_error( const string& what_arg);
Constructs an object of class underflow_error.
Example
// // exception.cpp // #include <iostream.h> #include <stdexcept> static void f() { throw runtime_error("a runtime error"); } int main () { // // By wrapping the body of main in a try-catch block // we can be assured that we'll catch all exceptions // in the exception hierarchy. You can simply catch // exception as is done below, or you can catch each // of the exceptions in which you have an interest. // try { f(); } catch (const exception& e) { cout << "Got an exception: " << e.what() << endl; } return 0; }
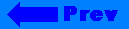
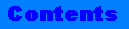
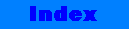
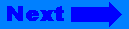
©Copyright 1996, Rogue Wave Software, Inc.