Personal tools
find_first_of

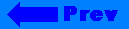
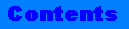
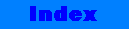
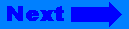
Click on the banner to return to the class reference home page.
find_first_of
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warnings
- See Also
Summary
Finds the first occurrence of any value from one sequence in another sequence.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class ForwardIterator1, class ForwardIterator2> ForwardIterator1 find_first_of (ForwardIterator1 first1, ForwardIterator1 last1, ForwardIterator2 first2, ForwardIterator2 last2); template <class ForwardIterator1, class ForwardIterator2, class BinaryPredicate> ForwardIterator1 find_first_of (ForwardIterator1 first1, ForwardIterator1 last1, ForwardIterator2 first2, ForwardIterator2 last2, BinaryPredicate pred);
Description
The find_first_of algorithm finds a the first occurrence of a value from a sequence, specified by first2, last2, in a sequence specified by first1, last1. The algorithm returns an iterator in the range [first1, last1) that points to the first matching element. If the first sequence [first1, last1) does not contain any of the values in the second sequence, find_first_of returns last1.
In other words, find_first_of returns the first iterator i in the [first1, last1)such that for some integer j in the range [first2, last2):the following conditions hold:
*i == *j, pred(*i,*j) == true.
Or find_first_of returns last1 if no such iterator is found.
Complexity
Two versions of the algorithm exist. The first uses the equality operator as the default binary predicate, and the second allows you to specify a binary predicate.
At most (last1 - first1)*(last2 - first2) applications of the corresponding predicate are done.
Example
// // find_f_o.cpp // #include <vector> #include <iterator> #include <algorithm> #include <iostream.h> int main() { typedef vector<int>::iterator iterator; int d1[10] = {0,1,2,2,3,4,2,2,6,7}; int d2[2] = {6,4}; // // Set up two vectors // vector<int> v1(d1,d1 + 10), v2(d2,d2 + 2); // // Try both find_first_of variants // iterator it1 = find_first_of(v1.begin(),v1.end(),v2.begin(),v2.end()); find_first_of(v1.begin(),v1.end(),v2.begin(),v2.end(), equal_to<int>()); // // Output results // cout << "For the vectors: "; copy(v1.begin(),v1.end(), ostream_iterator<int,char>(cout," " )); cout << " and "; copy(v2.begin(),v2.end(), ostream_iterator<int,char>(cout," " )); cout << endl << endl << "both versions of find_first_of point to: " << *it1; return 0; } Output : For the vectors: 0 1 2 2 3 4 2 2 6 7 and 6 4 both versions of find_first_of point to: 4
Warnings
If your compiler does not support default template parameters then you need to always supply the Allocator template argument. For instance you'll have to write:
vector<int, allocator<int> >
instead of:
vector<int>
See Also
Algorithms, adjacent_find, find, find_if, find_next, find_end
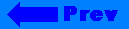
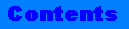
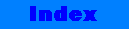
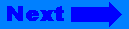
©Copyright 1996, Rogue Wave Software, Inc.