Personal tools
find_end

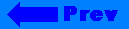
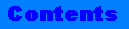
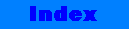
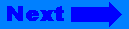
Click on the banner to return to the class reference home page.
find_end
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warnings
- See Also
Summary
Finds the last occurrence of a sub-sequence in a sequence.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class ForwardIterator1, class ForwardIterator2> ForwardIterator1 find_end(ForwardIterator1 first1, ForwardIterator1 last1, ForwardIterator2 first2, ForwardIterator2 last2); template <class Forward Iterator1, class ForwardIterator2, class BinaryPredicate> ForwardIterator1 find_end(ForwardIterator1 first1, ForwardIterator1 last1, ForwardIterator2 first2, ForwardIterator2 last2, BinaryPredicate pred);
Description
The find_end algorithm finds the last occurrence of a sub-sequence, indicated by [first2, last2), in a sequence, [first1,last1). The algorithm returns an iterator pointing to the first element of the found sub-sequence, or last1 if no match is found.
More precisely, the find_end algorithm returns the last iterator i in the range [first1, last1 - (last2-first2)) such that for any non-negative integer n < (last2-first2), the following corresponding conditions hold:
*(i+n) == *(first2+n), pred(*(i+n),*(first2+n)) == true.
Or returns last1 if no such iterator is found.
Two versions of the algorithm exist. The first uses the equality operator as the default binary predicate, and the second allows you to specify a binary predicate.
Complexity
At most (last2-first2)*(last1-first1-(last2-first2)+1) applications of the corresponding predicate are done.
Example
// // find_end.cpp // #include<vector> #include<iterator> #include<algorithm> #include<iostream.h> int main() { typedef vector<int>::iterator iterator; int d1[10] = {0,1,6,5,3,2,2,6,5,7}; int d2[4] = {6,5,0,0} // // Set up two vectors. // vector<int> v1(d1+0, d1+10), v2(d2+0, d2+2); // // Try both find_first_of variants. // iterator it1 = find_first_of (v1.begin(), v1.end(), v2.begin(), v2.end()); iterator it2 = find_first_of (v1.begin(), v1.end(), v2.begin(), v2.end(), equal_to<int>()); // // Try both find_end variants. // iterator it3 = find_end (v1.begin(), v1.end(), v2.begin(), v2.end()); iterator it4 = find_end (v1.begin(), v1.end(), v2.begin(), v2.end(), equal_to<int>()); // // Output results of find_first_of. // Iterator now points to the first element that matches one of // a set of values // cout << "For the vectors: "; copy (v1.begin(), v1.end(), ostream_iterator<int>(cout," ")); cout << " and "; copy (v2.begin(), v2.end(), ostream_iterator<int>(cout," ")); cout<< endl ,, endl << "both versions of find_first_of point to: " << *it1 << endl << "with first_of address = " << it1 << endl ; // //Output results of find_end. // Iterator now points to the first element of the last find //sub-sequence. // cout << endl << endl << "both versions of find_end point to: " << *it3 << endl << "with find_end address = " << it3 << endl ; return 0; } Output : For the vectors: 0 1 6 5 3 2 2 6 5 7 and 6 5 both versions of find_first_of point to: 6 with first_of address = 0x100005c0 both versions of find_end point to: 6 with find_end address = 0x100005d4
Warnings
If your compiler does not support default template parameters then you need to always supply the Allocator template argument. For instance you'll have to write:
vector<int, allocator<int> >
instead of:
vector<int>
See Also
Algorithms, find, find_if, adjacent_find
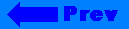
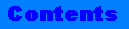
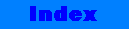
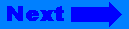
©Copyright 1996, Rogue Wave Software, Inc.