Personal tools
reverse_copy

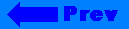
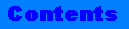
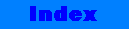
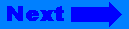
Click on the banner to return to the class reference home page.
reverse_copy
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warning
- See Also
Summary
Reverse the order of elements in a collection while copying them to a new collecton.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class BidirectionalIterator, class OutputIterator> OutputIterator reverse_copy (BidirectionalIterator first, BidirectionalIterator last, OutputIterator result);
Description
The reverse_copy algorithm copies the range [first, last) to the range [result, result + (last - first)) such that for any non- negative integer i < (last - first), the following assignment takes place:
*(result + (last - first) -i) = *(first + i)
reverse_copy returns result + (last - first). The ranges [first, last) and [result, result + (last - first)) must not overlap.
Complexity
reverse_copy performs exactly (last - first) assignments.
Example
// // reverse.cpp // #include <algorithm> #include <vector> #include <iostream.h> int main () { // // Initialize a vector with an array of integers. // int arr[10] = { 1,2,3,4,5,6,7,8,9,10 }; vector<int> v(arr+0, arr+10); // // Print out elements in original (sorted) order. // cout << "Elements before reverse: " << endl << " "; copy(v.begin(), v.end(), ostream_iterator<int,char>(cout," ")); cout << endl << endl; // // Reverse the ordering. // reverse(v.begin(), v.end()); // // Print out the reversed elements. // cout << "Elements after reverse: " << endl << " "; copy(v.begin(), v.end(), ostream_iterator<int,char>(cout," ")); cout << endl << endl; cout << "A reverse_copy to cout: " << endl << " "; reverse_copy(v.begin(), v.end(), ostream_iterator<int,char>(cout, " ")); cout << endl; return 0; } Output : Elements before reverse: 1 2 3 4 5 6 7 8 9 10 Elements after reverse: 10 9 8 7 6 5 4 3 2 1 A reverse_copy to cout: 1 2 3 4 5 6 7 8 9 10
Warning
If your compiler does not support default template parameters, then you need to always supply the Allocator template argument. For instance, you will need to write :
vector<int, allocator<int> >
instead of :
vector<int>
See Also
reverse
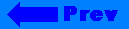
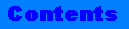
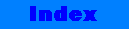
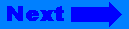
©Copyright 1996, Rogue Wave Software, Inc.