Personal tools
ostream_iterator

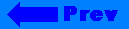
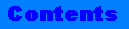
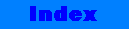
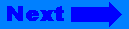
Click on the banner to return to the class reference home page.
ostream_iterator
Iterator
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors
- Destructor
- Operators
- Example
- Warning
- See Also
Summary
Stream iterators provide iterator capabilities for ostreams and istreams. They allow generic algorithms to be used directly on streams.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | |
char_type ostream_type traits_type value_type |
Member Functions |
operator*() operator++() operator=() |
Synopsis
#include <ostream> template <class T, class charT, class traits = char_traits<charT> > class ostream_iterator : public iterator<output_iterator_tag,void,void>;
Description
Stream iterators provide the standard iterator interface for input and output streams.
The class ostream_iterator writes elements to an output stream. If you use the constructor that has a second, char * argument, then that string will be written after every element . (The string must be null-terminated.) Since an ostream iterator is an output iterator, it is not possible to get an element out of the iterator. You can only assign to it.
Interface
template <class T, class charT, class traits = char_traits<charT> > class ostream_iterator : public iterator<output_iterator_tag,void,void> { public: typedef T value_type; typedef charT char_type; typedef traits traits_type; typedef basic_ostream<charT,traits> ostream_type; ostream_iterator(ostream&); ostream_iterator (ostream&, const char*); ostream_iterator (const ostream_iterator<T,charT,char_traits<charT> >&); ~ostream_itertor (); ostream_iterator<T,charT,char_traits<charT> >& operator=(const T&); ostream_iterator<T,charT,char_traits<charT> >& operator* () const; ostream_iterator<T,charT,char_traits<charT> >& operator++ (); ostream_iterator<T,charT,char_traits<charT> > operator++ (int); };
Types
value_type;
Type of value to stream in.
char_type;
Type of character the stream is built on.
traits_type;
Traits used to build the stream.
ostream_type;
Type of stream this iterator is constructed on.
Constructors
ostream_iterator (ostream& s);
Construct an ostream_iterator on the given stream.
ostream_iterator (ostream& s, const char* delimiter);
Construct an ostream_iterator on the given stream. The null terminated string delimitor is written to the stream after every element.
ostream_iterator (const ostream_iterator<T>& x);
Copy constructor.
Destructor
~ostream_iterator ();
Destructor
Operators
const T& operator= (const T& value);
Shift the value T onto the output stream.
const T& ostream_iterator<T>& operator* (); ostream_iterator<T>& operator++(); ostream_iterator<T> operator++ (int);
These operators all do nothing. They simply allow the iterator to be used in common constructs.
Example
#include <iterator> #include <numeric> #include <deque> #include <iostream.h> int main () { // // Initialize a vector using an array. // int arr[4] = { 3,4,7,8 }; int total=0; deque<int> d(arr+0, arr+4); // // stream the whole vector and a sum to cout // copy(d.begin(),d.end()-1, ostream_iterator<int,char>(cout," + ")); cout << *(d.end()-1) << " = " << accumulate(d.begin(),d.end(),total) << endl; return 0; }
Warning
If your compiler does not support default template parameters, then you need to always supply the Allocator template argument. For instance, you will need to write :
deque<int, allocator<int> >
instead of :
deque<int>
See Also
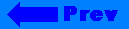
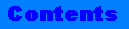
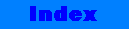
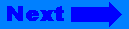
©Copyright 1996, Rogue Wave Software, Inc.