Personal tools
istream_iterator

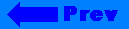
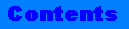
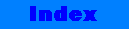
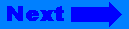
Click on the banner to return to the class reference home page.
istream_iterator
Iterators
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors
- Destructors
- Operators
- Non-member Operators
- Example
- Warning
- See Also
Summary
Stream iterator that provides iterator capabilities for istreams. This iterator allows generic algorithms to be used directly on streams.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | |
char_type istream_type traits_type value_type |
Member Functions |
operator operator!=() operator*() ()">operator->() operator==() |
Synopsis
#include <iterator> template <class T, class charT, class traits = ios_traits<charT>, class Distance = ptrdiff_t> class istream_iterator : public iterator<input_iterator_tag, T,Distance>;
Description
Stream iterators provide the standard iterator interface for input and output streams.
The class istream_iterator reads elements from an input stream (using operator >>). A value of type T is retrieved and stored when the iterator is constructed and each time operator++ is called. The iterator will be equal to the end-of-stream iterator value if the end-of-file is reached. Use the constructor with no arguments to create an end-of-stream iterator. The only valid use of this iterator is to compare to other iterators when checking for end of file. Do not attempt to dereference the end-of-stream iterator; it plays the same role as the past-the-end iterator provided by the end() function of containers. Since an istream_iterator is an input iterator, you cannot assign to the value returned by dereferencing the iterator. This also means that istream_iterators can only be used for single pass algorithms.
Since a new value is read every time the operator++ is used on an istream_iterator, that operation is not equality-preserving. This means that i == j does not mean that ++i == ++j (although two end-of-stream iterators are always equal).
Interface
template <class T, class charT, class traits = ios_traits<charT> class Distance = ptrdiff_t> class istream_iterator : public iterator<input_iterator_tag, T, Distance> { public: typedef T value_type; typedef charT char_type; typedef traits traits_type; typedef basic_istream<charT,traits> istream_type; istream_iterator(); istream_iterator (istream_type&); istream_iterator (const stream_iterator<T,charT,traits,Distance>&); ~istream_itertor (); const T& operator*() const; const T* operator ->() const; istream_iterator <T,charT,traits,Distance>& operator++(); istream_iterator <T,charT,traits,Distance> operator++ (int) }; // Non-member Operators template <class T, class charT, class traits, class Distance> bool operator==(const istream_iterator<T,charT,traits,Distance>&, const istream_iterator<T,charT,traits,Distance>&); template <class T, class charT, class traits, class Distance> bool operator!=(const istream_iterator<T,charT,traits,Distance>&, const istream_iterator<T,charT,traits,Distance>&);
Types
value_type;
Type of value to stream in.
char_type;
Type of character the stream is built on.
traits_type;
Traits used to build the stream.
istream_type;
Type of stream this iterator is constructed on.
Constructors
istream_iterator();
Construct an end-of-stream iterator. This iterator can be used to compare against an end-of-stream condition. Use it to provide end iterators to algorithms
istream_iterator(istream& s);
Construct an istream_iterator on the given stream.
istream_iterator(const istream_iterator& x);
Copy constructor.
Destructors
~istream_iterator();
Destructor.
Operators
const T& operator*() const;
Return the current value stored by the iterator.
const T* operator->() const;
Return a pointer to the current value stored by the iterator.
istream_iterator& operator++() istream_iterator operator++(int)
Retrieve the next element from the input stream.
Non-member Operators
bool operator==(const istream_iterator<T,charT,traits,Distance>& x, const istream_iterator<T,charT,traits,Distance>& y)
Equality operator. Returns true if x is the same as y.
bool operator!=(const istream_iterator<T,charT,traits,Distance>& x, const istream_iterator<T,charT,traits,Distance>& y)
Inequality operator. Returns true if x is not the same as y.
Example
// // io_iter.cpp // #include <iterator> #include <vector> #include <numeric> #include <iostream.h> int main () { vector<int> d; int total = 0; // // Collect values from cin until end of file // Note use of default constructor to get ending iterator // cout << "Enter a sequence of integers (eof to quit): " ; copy(istream_iterator<int,char>(cin), istream_iterator<int,char>(), inserter(d,d.begin())); // // stream the whole vector and the sum to cout // copy(d.begin(),d.end()-1, ostream_iterator<int,char>(cout," + ")); if (d.size()) cout << *(d.end()-1) << " = " << accumulate(d.begin(),d.end(),total) << endl; return 0; }
Warning
If your compiler does not support default template parameters, then you will need to always supply the Allocator template argument. And you'll have to provide all parameters to the istream_iterator template. For instance, you'll have to write :
vector<int, allocator<int> >
instead of :
vector<int>
See Also
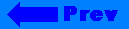
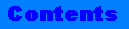
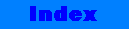
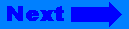
©Copyright 1996, Rogue Wave Software, Inc.