Personal tools
istreambuf_iterator

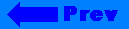
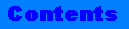
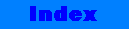
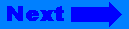
Click on the banner to return to the class reference home page.
istreambuf_iterator
istreambuf_iteratorinput_iterator
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Nested Class Proxy
- Constructors
- Member Operators
- Public Member Function
- Non Member Functions
- Examples
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | |
char_type int_type istream_type streambuf_type |
traits_type |
Member Functions |
equal() operator*() operator++() operator==() |
Synopsis
#include <streambuf> template<class charT, class traits = char_traits<charT> > class istreambuf_iterator : public input_iterator
Description
The template class istreambuf_iterator reads successive characters from the stream buffer for which it was constructed. operator* provides access to the current input character, if any, and operator++ advances to the next input character. If the end of stream is reached, the iterator becomes equal to the end of stream iterator value, which is constructed by the default constructor, istreambuf_iterator(). An istreambuf_iterator object can be used only for one-pass-algorithms.
Interface
template<class charT, class traits = char_traits<charT> > class istreambuf_iterator : public input_iterator { public: typedef charT char_type; typedef typename traits::int_type int_type; typedef traits traits_type; typedef basic_streambuf<charT, traits> streambuf_type; typedef basic_istream<charT, traits> istream_type; class proxy; istreambuf_iterator() throw(); istreambuf_iterator(istream_type& s) throw(); istreambuf_iterator(streambuf_type *s) throw(); istreambuf_iterator(const proxy& p) throw(); char_type operator*(); istreambuf_iterator<charT, traits>& operator++(); proxy operator++(int); bool equal(istreambuf_iterator<charT, traits>& b); }; template<class charT, class traits> bool operator==(istreambuf_iterator<charT, traits>& a, istreambuf_iterator<charT, traits>& b);
Types
char_type
The type char_type is a synonym for the template parameter charT.
int_type
The type int_type is a synonym of type traits::in_type.
istream_type
The type istream_type is an instantiation of class basic_istream on types charT and traits:
typedef basic_istream<charT, traits> istream_type;
streambuf_type
The type streambuf_type is an instantiation of class basic_streambuf on types charT and traits:
typedef basic_streambuf<charT, traits> streambuf_type;
traits_type
The type traits_type is a synonym for the template parameter traits.
Nested Class Proxy
Class istreambuf_iterator<charT,traits>::proxy provides a temporary placeholder as the return value of the post-increment operator. It keeps the character pointed to by the previous value of the iterator for some possible future access.
Constructors
istreambuf_iterator() throw();
Constructs the end of stream iterator.
istreambuf_iterator(istream_type& s) throw();
Constructs an istreambuf_iterator that inputs characters using the basic_streambuf object pointed to by s.rdbuf(). If s.rdbuf() is a null pointer, the istreambuf_iterator is the end-of-stream iterator.
istreambuf_iterator(streambuf_type *s) throw();
Constructs an istreambuf_iterator that inputs characters using the basic_streambuf object pointed at by s. If s is a null pointer, the istreambuf_iterator is the end-of-stream iterator.
istreambuf_iterator(const proxy& p) throw();
Constructs an istreambuf_iterator that uses the basic_streambuf object embedded in the proxy object.
Member Operators
char_type operator*();
Returns the character pointed at by the input sequence of the attached stream buffer. If no character is available, the iterator becomes equal to the end-of-stream iterator.
istreambuf_iterator<charT, traits>& operator++();
Increments the input sequence of the attached stream buffer to point to the next character. If the current character is the last one, the iterator becomes equal to the end-of-stream iterator.
proxy operator++(int);
Increments the input sequence of the attached stream buffer to point to the next character. If the current character is the last one, the iterator becomes equal to the end-of-stream iterator. The proxy object returned contains the character pointed at before carrying out the post-increment operator.
Public Member Function
bool equal(istreambuf_iterator<charT, traits>& b);
Returns true if and only if both iterators are at end of stream, or neither is at end of stream, regardless of what stream buffer object they are using.
Non Member Functions
template<class charT, class traits> bool operator==(istreambuf_iterator<charT, traits>& a, istreambuf_iterator<charT, traits>& b);
Returns a.equal(b).
Examples
// // stdlib/examples/manual/istreambuf_iterator.cpp // #include<iostream> #include<fstream> void main ( ) { using namespace std; // open the file is_iter.out for reading and writing ofstream out("is_iter.out", ios_base::out | ios_base::in ); // output the example sentence into the file out << "Ceci est un simple example pour demontrer le" << endl; out << "fonctionement de istreambuf_iterator"; // seek to the beginning of the file out.seekp(0); // construct an istreambuf_iterator pointing to // the ofstream object underlying stream buffer istreambuf_iterator<char> iter(out.rdbuf()); // construct an end of stream iterator istreambuf_iterator<char> end_of_stream_iterator; cout << endl; // output the content of the file while( !iter.equal(end_of_stream_iterator) ) // use both operator++ and operator* cout << *iter++; cout << endl; }
See Also
basic_streambuf(3C++), basic_istream(3C++), ostreambuf_iterator(3C++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Section 24.4.3
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
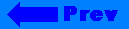
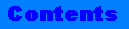
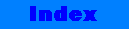
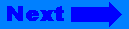
©Copyright 1996, Rogue Wave Software, Inc.