Personal tools
generate, generate_n

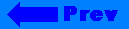
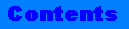
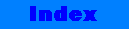
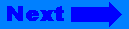
Click on the banner to return to the class reference home page.
generate, generate_n
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warnings
- See Also
Summary
Initialize a container with values produced by a value-generator class.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class ForwardIterator, class Generator> void generate(ForwardIterator first, ForwardIterator last, Generator gen); template <class OutputIterator, class Size, class Generator> void generate_n(OutputIterator first, Size n, Generator gen);
Description
A value-generator function returns a value each time it is invoked. The algorithms generate and generate_n initialize (or reinitialize) a sequence by assigning the return value of the generator function gen to all the elements designated by iterators in the range [first, last) or [first, first + n). The function gen takes no arguments. (gen can be a function or a class with an operator () defined that takes no arguments.)
generate_n assumes that there are at least n elements following first, unless first is an insert iterator.
Complexity
The generate and generate_n algorithms invoke gen and assign its return value exactly last - first (or n) times.
Example
// // generate.cpp // #include <algorithm> #include <vector> #include <iostream.h> // Value generator simply doubles the current value // and returns it template <class T> class generate_val { private: T val_; public: generate_val(const T& val) : val_(val) {} T& operator()() { val_ += val_; return val_; } }; int main() { int d1[4] = {1,2,3,4}; generate_val<int> gen(1); // Set up two vectors vector<int> v1(d1,d1 + 4), v2(d1,d1 + 4); // Set up one empty vector vector<int> v3; // Generate values for all of v1 generate(v1.begin(),v1.end(),gen); // Generate values for first 3 of v2 generate_n(v2.begin(),3,gen); // Use insert iterator to generate 5 values for v3 generate_n(back_inserter(v3),5,gen); // Copy all three to cout ostream_iterator<int,char> out(cout," "); copy(v1.begin(),v1.end(),out); cout << endl; copy(v2.begin(),v2.end(),out); cout << endl; copy(v3.begin(),v3.end(),out); cout << endl; // Generate 3 values for cout generate_n(ostream_iterator<int>(cout," "),3,gen); cout << endl; return 0; } Output : 2 4 8 16 2 4 8 4 2 4 8 16 32 2 4 8
Warnings
If your compiler does not support default template parameters, then you need to always supply the Allocator template argument. For instance, you'll have to write:
vector<int, allocator<int> >
instead of :
vector<int>
See Also
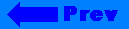
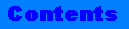
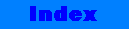
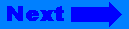
©Copyright 1996, Rogue Wave Software, Inc.