Personal tools
Function Objects

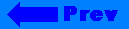
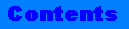
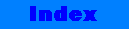
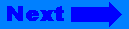
Click on the banner to return to the class reference home page.
Function Objects
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Example
- Warnings
- See Also
Summary
Objects with an operator() defined. Function objects are used in place of pointers to functions as arguments to templated algorithms.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include<functional> // typedefs template <class Arg, class Result> struct unary_function; template <class Arg1, class Arg2, class Result> struct binary_function;
Description
Function objects are objects with an operator() defined. They are important for the effective use of the standard library's generic algorithms, because the interface for each algorithmic template can accept either an object with an operator() defined, or a pointer to a function. The Standard C++ Library provides both a standard set of function objects, and a pair of classes that you can use as the base for creating your own function objects.
Function objects that take one argument are called unary function objects. Unary function objects are required to provide the typedefs argument_type and result_type. Similarly, function objects that take two arguments are called binary function objects and, as such, are required to provide the typedefs first_argument_type, second_argument_type, and result_type.
The classes unary_function and binary_function make the task of creating templated function objects easier. The necessary typedefs for a unary or binary function object are provided by inheriting from the appropriate function object class.
The function objects provided by the standard library are listed below, together with a brief description of their operation. This class reference also includes an alphabetic entry for each function.
Name |
Operation |
arithmetic functions |
|
plus |
addition x + y |
minus |
subtraction x - y |
multiplies |
multiplication x * y |
divides |
division x / y |
modulus |
remainder x % y |
negate |
negation - x |
comparison functions |
|
equal_to |
equality test x == y |
not_equal_to |
inequality test x != y |
greater |
greater comparison x > y |
less |
less-than comparison x < y |
greater_equal |
greater than or equal comparison x >= y |
less_equal |
less than or equal comparison x <= y |
logical functions |
|
logical_and |
logical conjunction x && y |
logical_or |
logical disjunction x || y |
logical_not |
logical negation ! x |
Interface
template <class Arg, class Result> struct unary_function{ typedef Arg argument_type; typedef Result result_type; }; template <class Arg1, class Arg2, class Result> struct binary_function{ typedef Arg1 first_argument_type; typedef Arg2 second_argument_type; typedef Result result_type; }; // Arithmetic Operations template<class T> struct plus : binary_function<T, T, T> { T operator() (const T&, const T&) const; }; template <class T> struct minus : binary_function<T, T, T> { T operator() (const T&, const T&) const; }; template <class T> struct multiplies : binary_function<T, T, T> { T operator() (const T&, const T&) const; }; template <class T> struct divides : binary_function<T, T, T> { T operator() (const T&, const T&) const; }; template <class T> struct modulus : binary_function<T, T, T> { T operator() (const T&, const T&) const; }; template <class T> struct negate : unary_function<T, T> { T operator() (const T&) const; }; // Comparisons template <class T> struct equal_to : binary_function<T, T, bool> { bool operator() (const T&, const T&) const; }; template <class T> struct not_equal_to : binary_function<T, T, bool> { bool operator() (const T&, const T&) const; }; template <class T> struct greater : binary_function<T, T, bool> { bool operator() (const T&, const T&) const; }; template <class T> struct less : binary_function<T, T, bool> { bool operator() (const T&, const T&) const; }; template <class T> struct greater_equal : binary_function<T, T, bool> { bool operator() (const T&, const T&) const; }; template <class T> struct less_equal : binary_function<T, T, bool> { bool operator() (const T&, const T&) const; }; // Logical Comparisons template <class T> struct logical_and : binary_function<T, T, bool> { bool operator() (const T&, const T&) const; }; template <class T> struct logical_or : binary_function<T, T, bool> { bool operator() (const T&, const T&) const; }; template <class T> struct logical_not : unary_function<T, T, bool> { bool operator() (const T&, const T&) const; };
Example
// // funct_ob.cpp // #include<functional> #include<deque> #include<vector> #include<algorithm> #include <iostream.h> //Create a new function object from unary_function template<class Arg> class factorial : public unary_function<Arg, Arg> { public: Arg operator()(const Arg& arg) { Arg a = 1; for(Arg i = 2; i <= arg; i++) a *= i; return a; } }; int main() { //Initialize a deque with an array of ints int init[7] = {1,2,3,4,5,6,7}; deque<int> d(init, init+7); //Create an empty vector to store the factorials vector<int> v((size_t)7); //Transform the numbers in the deque to their factorials and // store in the vector transform(d.begin(), d.end(), v.begin(), factorial<int>()); //Print the results cout << "The following numbers: " << endl << " "; copy(d.begin(),d.end(),ostream_iterator<int,char>(cout," ")); cout << endl << endl; cout << "Have the factorials: " << endl << " "; copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); return 0; } Output : The following numbers: 1 2 3 4 5 6 7 Have the factorials: 1 2 6 24 120 720 5040
Warnings
If your compiler does not support default template parameters, then you need to always supply the Allocator template argument. For instance, you'll have to write :
vector<int, allocator<int> > and deque<int, allocator<int> >
instead of :
vector<int> and deque<int>
See Also
binary_function, unary_function
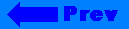
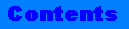
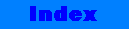
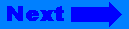
©Copyright 1996, Rogue Wave Software, Inc.