Personal tools
Negators

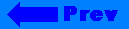
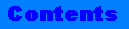
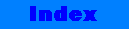
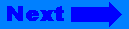
Click on the banner to return to the class reference home page.
Negators
Function Object
Summary
Function adaptors and function objects used to reverse the sense of predicate function objects.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <functional> template <class Predicate> class unary_negate; template <class Predicate> unary_negate<Predicate> not1(const Predicate&); template <class Predicate> class binary_negate; template <class Predicate> binary_negate<Predicate> not2(const Predicate&);
Description
Negators not1 and not2 are functions that take predicate function objects as arguments and return predicate function objects with the opposite sense. Negators work only with function objects defined as subclasses of the classes unary_function and binary_function. not1 accepts and returns unary predicate function objects. not2 accepts and returns binary predicate function objects.
unary_negate and binary_negate are function object classes that provide return types for the negators, not1 and not2.
Interface
template <class Predicate> class unary_negate : public unary_function<typename Predicate::argument_type, bool> { public: typedef typename unary_function<typename Predicate::argument_type, bool>::argument_type argument_type; typedef typename unary_function<typename Predicate::argument_type, bool>::result_type result_type; explicit unary_negate (const Predicate&); bool operator() (const argument_type&) const; }; template<class Predicate> unary_negate <Predicate> not1 (const Predicate&); template<class Predicate> class binary_negate : public binary_function<typename Predicate::first_argument_type, typename Predicate::second_argument_type, bool> { public: typedef typename binary_function<typename Predicate::first_argument_type, typename Predicate::second_argument_type, bool>::second_argument_type second_argument_type; typedef typename binary_function<typename Predicate::first_argument_type, typename Predicate::second_argument_type, bool>::first_argument_type first_argument_type; typedef typename binary_function<typename Predicate::first_argument_type, typename Predicate::second_argument_type, bool>::result_type result_type; explicit binary_negate (const Predicate&); bool operator() (const first_argument_type&, const second_argument_type&) const; }; template <class Predicate> binary_negate<Predicate> not2 (const Predicate&);
Example
// // negator.cpp // #include<functional> #include<algorithm> #include <iostream.h> //Create a new predicate from unary_function template<class Arg> class is_odd : public unary_function<Arg, bool> { public: bool operator()(const Arg& arg1) const { return (arg1 % 2 ? true : false); } }; int main() { less<int> less_func; // Use not2 on less cout << (less_func(1,4) ? "TRUE" : "FALSE") << endl; cout << (less_func(4,1) ? "TRUE" : "FALSE") << endl; cout << (not2(less<int>())(1,4) ? "TRUE" : "FALSE") << endl; cout << (not2(less<int>())(4,1) ? "TRUE" : "FALSE") << endl; //Create an instance of our predicate is_odd<int> odd; // Use not1 on our user defined predicate cout << (odd(1) ? "TRUE" : "FALSE") << endl; cout << (odd(4) ? "TRUE" : "FALSE") << endl; cout << (not1(odd)(1) ? "TRUE" : "FALSE") << endl; cout << (not1(odd)(4) ? "TRUE" : "FALSE") << endl; return 0; } Output : TRUE FALSE FALSE TRUE TRUE FALSE FALSE TRUE
See Also
Algorithms, binary_function, Function Objects, unary_function
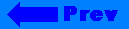
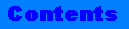
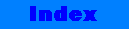
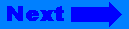
©Copyright 1996, Rogue Wave Software, Inc.