Personal tools
19.3 Example Program

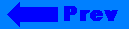



Click on the banner to return to the user guide home page.
19.3 Example Program
This program illustrates the use of auto_ptr to ensure that pointers held in a vector are deleted when they are removed. Often, we might want to hold pointers to strings, since the strings themselves may be quite large and we'll be copying them when we put them into the vector. Particularly in contrast to a string, an auto_ptr is quite small: hardly bigger than a pointer. (Note that the program runs as is because the vector includes memory.)

#include <vector> #include <memory> #include <string> int main() { { // First the wrong way vector<string*> v; v.insert(v.begin(), new string("Florence")); v.insert(v.begin(), new string("Milan")); v.insert(v.begin(), new string("Venice")); // Now remove the first element v.erase(v.begin()); // Whoops, memory leak // string("Venice") was removed, but not deleted // We were supposed to handle that ourselves } { // Now the right way vector<auto_ptr<string> > v; v.insert(v.begin(), auto_ptr<string>(new string("Florence"))); v.insert(v.begin(), auto_ptr<string>(new string("Milan"))); v.insert(v.begin(), auto_ptr<string>(new string("Venice"))); // Everything is fine since auto_ptrs transfer ownership of // their pointers when copied // Now remove the first element v.erase(v.begin()); // Success // When auto_ptr(string("Venice")) is erased (and destroyed) // string("Venice") is deleted } return 0; }
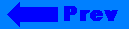



©Copyright 1996, Rogue Wave Software, Inc.