Personal tools
8.3 Example Program: - A Spelling Checker

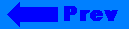



Click on the banner to return to the user guide home page.
8.3 Example Program: - A Spelling Checker

A simple example program that uses a set is a spelling checker. The checker takes as arguments two input streams; the first representing a stream of correctly spelled words (that is, a dictionary), and the second a text file. First, the dictionary is read into a set. This is performed using a copy() and an input stream iterator, copying the values into an inserter for the dictionary. Next, words from the text are examined one by one, to see if they are in the dictionary. If they are not, then they are added to a set of misspelled words. After the entire text has been examined, the program outputs the list of misspelled words.
void spellCheck (istream & dictionary, istream & text) { typedef set <string, less<string> > stringset; stringset words, misspellings; string word; istream_iterator<string, ptrdiff_t> dstream(dictionary), eof; // first read the dictionary copy (dstream, eof, inserter(words, words.begin())); // next read the text while (text >> word) if (! words.count(word)) misspellings.insert(word); // finally, output all misspellings cout << "Misspelled words:" << endl; copy (misspellings.begin(), misspellings.end(), ostream_iterator<string>(cout, "\n")); }
An improvement would be to suggest alternative words for each misspelling. There are various heuristics that can be used to discover alternatives. The technique we will use here is to simply exchange adjacent letters. To find these, a call on the following function is inserted into the loop that displays the misspellings.
void findMisspell(stringset & words, string & word) { for (int I = 1; I < word.length(); I++) { swap(word[I-1], word[I]); if (words.count(word)) cout << "Suggestion: " << word << endl; // put word back as before swap(word[I-1], word[I]); } }
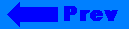



©Copyright 1996, Rogue Wave Software, Inc.