Personal tools
8.4 The bitset Abstraction

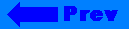
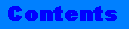
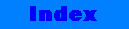
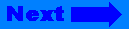
Click on the banner to return to the user guide home page.
8.4 The bitset Abstraction
A bitset is really a cross between a set and a vector. Like the vector abstraction vector<bool>, the abstraction represents a set of binary (0/1 bit) values. However, set operations can be performed on bitsets using the logical bit-wise operators. The class bitset does not provide any iterators for accessing elements.
8.4.1 Include Files
#include <bitset>
8.4.2 Declaration and Initialization of bitset
A bitset is a template class abstraction. The template argument is not, however, a type, but an integer value. The value represents the number of bits the set will contains.
bitset<126> bset_one; // create a set of 126 bits
An alternative technique permits the size of the set to be specified as an argument to the constructor. The actual size will be the smaller of the value used as the template argument and the constructor argument. This technique is useful when a program contains two or more bit vectors of differing sizes. Consistently using the larger size for the template argument means that only one set of methods for the class will be generated. The actual size, however, will be determined by the constructor.
bitset<126> bset_two(100); // this set has only 100 elements
A third form of constructor takes as argument a string of 0 and 1 characters. A bitset is created that has as many elements as are characters in the string, and is initialized with the values from the string.
bitset<126> small_set("10101010"); // this set has 8 elements
8.4.3 Accessing and Testing Elements
An individual bit in the bitset can be accessed using the subscript operation. Whether the bit is one or not can be determined using the member function test(). Whether any bit in the bitset is "on" is tested using the member function any(), which yields a boolean value. The inverse of any() is returned by the member function none().
bset_one[3] = 1; if (bset_one.test(4)) cout << "bit position 4 is set" << endl; if (bset_one.any()) cout << "some bit position is set" << endl; if (bset_one.none()) cout << "no bit position is set" << endl;
The function set() can be used to set a specific bit. bset_one.set(I) is equivalent to bset_one[I] = true. Invoking the function without any arguments sets all bit positions to true. The function reset() is similar, and sets the indicated positions to false (sets all positions to false if invoked with no argument). The function flip() flips either the indicated position, or all positions if no argument is provided. The function flip() is also provided as a member function for the individual bit references.
bset_one.flip(); // flip the entire set bset_one.flip(12); // flip only bit 12 bset_one[12].flip(); // reflip bit 12
The member function size() returns the size of the bitset, while the member function count() yields the number of bits that are set.
8.4.4 Set operations
Set operations on bitsets are implemented using the bit-wise operators, in a manner analogous to the way in which the same operators act on integer arguments.
The negation operator (operator ~) applied to a bitset returns a new bitset containing the inverse of elements in the argument set.
The intersection of two bitsets is formed using the and operator (operator &). The assignment form of the operator can be used. In the assignment form, the target becomes the disjunction of the two sets.
bset_three = bset_two & bset_four; bset_five &= bset_three;
The union of two sets is formed in a similar manner using the or operator (operator |). The exclusive-or is formed using the bit-wise exclusive or operator (operator ^).
The left and right shift operators (operator << and >>) can be used to shift a bitset left or right, in a manner analogous to the use of these operators on integer arguments. If a bit is shifted left by an integer value n, then the new bit position I is the value of the former I-n. Zeros are shifted into the new positions.
8.4.5 Conversions
The member function to_ulong() converts a bitset into an unsigned long. It is an error to perform this operation on a bitset containing more elements than will fit into this representation.
The member function to_string() converts a bitset into an object of type string. The string will have as many characters as the bitset. Each zero bit will correspond to the character 0, while each one bit will be represented by the character 1.
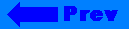
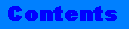
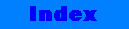
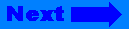
©Copyright 1996, Rogue Wave Software, Inc.