Personal tools
set_difference

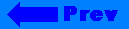
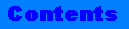
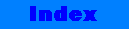
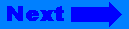
Click on the banner to return to the class reference home page.
set_difference
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warning
- See Also
Summary
Basic set operation for sorted sequences.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class InputIterator1, class InputIterator2, class OutputIterator> OutputIterator set_difference (InputIterator1 first1, InputIterator1 last1, InputIterator2 first2, InputIterator2 last2, OutputIterator result); template <class InputIterator1, class InputIterator2, class OutputIterator, class Compare> OutputIterator set_difference (InputIterator1 first1, InputIterator1 last1, InputIterator2 first2, InputIterator2 last2, OutputIterator result, Compare comp);
Description
The set_difference algorithm constructs a sorted difference that includes copies of the elements that are present in the range [first1, last1) but are not present in the range [first2, last2). It returns the end of the constructed range.
As an example, assume we have the following two sets:
1 2 3 4 5
and
3 4 5 6 7
The result of applying set_difference is the set:
1 2
The result of set_difference is undefined if the result range overlaps with either of the original ranges.
set_difference assumes that the ranges are sorted using the default comparison operator less than (<), unless an alternative comparison operator (comp) is provided.
Use the set_symetric_difference algorithm to return a result that contains all elements that are not in common between the two sets.
Complexity
At most ((last1 - first1) + (last2 - first2)) * 2 -1 comparisons are performed.
Example
// // set_diff.cpp // #include <algorithm> #include <set> #include <iostream.h> int main() { //Initialize some sets int a1[10] = {1,2,3,4,5,6,7,8,9,10}; int a2[6] = {2,4,6,8,10,12}; set<int, less<int> > all(a1, a1+10), even(a2, a2+6), odd; //Create an insert_iterator for odd insert_iterator<set<int, less<int> > > odd_ins(odd, odd.begin()); //Demonstrate set_difference cout << "The result of:" << endl << "{"; copy(all.begin(),all.end(), ostream_iterator<int,char>(cout," ")); cout << "} - {"; copy(even.begin(),even.end(), ostream_iterator<int,char>(cout," ")); cout << "} =" << endl << "{"; set_difference(all.begin(), all.end(), even.begin(), even.end(), odd_ins); copy(odd.begin(),odd.end(), ostream_iterator<int,char>(cout," ")); cout << "}" << endl << endl; return 0; } Output : The result of: {1 2 3 4 5 6 7 8 9 10 } - {2 4 6 8 10 12 } = {1 3 5 7 9 }
Warning
If your compiler does not support default template parameters, then you need to always supply the Compare template argument and the Allocator template argument. For instance, you will need to write :
set<int, less<int> allocator<int> >
instead of :
set<int>
See Also
includes, set, set_union, set_intersection, set_symmetric_difference
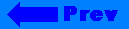
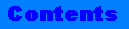
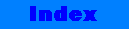
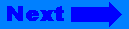
©Copyright 1996, Rogue Wave Software, Inc.