Personal tools
adjacent_find

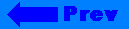
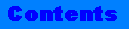
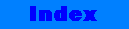
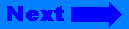
Click on the banner to return to the class reference home page.
adjacent_find
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warning
- See Also
Summary
Find the first adjacent pair of elements in a sequence that are equivalent.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class ForwardIterator> ForwardIterator adjacent_find(ForwardIterator first, ForwardIterator last); template <class ForwardIterator, class BinaryPredicate> ForwardIterator adjacent_find(ForwardIterator first, ForwardIterator last, BinaryPredicate pred);
Description
There are two versions of the adjacent_find algorithm. The first finds equal adjacent elements in the sequence defined by iterators first and last and returns an iterator i pointing to the first of the equal elements. The second version lets you specify your own binary function to test for a condition. It returns an iterator i pointing to the first of the pair of elements that meet the conditions of the binary function. In other words, adjacent_find returns the first iterator i such that both i and i + 1 are in the range [first, last) for which one of the following conditions holds:
*i == *(i + 1)
or
pred(*i,*(i + 1)) == true
If adjacent_find does not find a match, it returns last.
Complexity
adjacent_find performs exactly find(first,last,value) - first applications of the corresponding predicate.
Example
// // find.cpp // #include <vector> #include <algorithm> #include <iostream.h> int main() { typedef vector<int>::iterator iterator; int d1[10] = {0,1,2,2,3,4,2,2,6,7}; // Set up a vector vector<int> v1(d1,d1 + 10); // Try find iterator it1 = find(v1.begin(),v1.end(),3); // Try find_if iterator it2 = find_if(v1.begin(),v1.end(),bind1st(equal_to<int>(),3)); // Try both adjacent_find variants iterator it3 = adjacent_find(v1.begin(),v1.end()); iterator it4 = adjacent_find(v1.begin(),v1.end(),equal_to<int>()); // Output results cout << *it1 << " " << *it2 << " " << *it3 << " " << *it4 << endl; return 0; } Output : 3 3 2 2
Warning
If your compiler does not support default template parameters then you need to always supply the Allocator template argument. For instance you'll have to write:
vector<int,allocator<int> >
instead of:
vector<int>
See Also
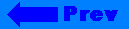
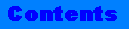
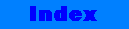
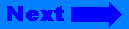
©Copyright 1996, Rogue Wave Software, Inc.