Personal tools
mismatch

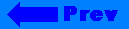
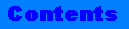
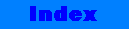
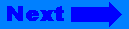
Click on the banner to return to the class reference home page.
mismatch
Algorithm
Summary
Compares elements from two sequences and returns the first two elements that don't match each other.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class InputIterator1, class InputIterator2> pair<InputIterator1,InputIterator2> mismatch(InputIterator1 first1, InputIterator1 last1, InputIterator2 first2); template <class InputIterator1, class InputIterator2, class BinaryPredicate> pair<InputIterator1, Inputiterator2> mismatch(InputIterator first1, InputIterator1 last1, InputIterator2 first2, BinaryPredicate binary_pred);
Description
The mismatch algorithm compares members of two sequences and returns two iterators (i and j) that point to the first location in each sequence where the sequences differ from each other. Notice that the algorithm denotes both a starting position and an ending position for the first sequence, but denotes only a starting position for the second sequence. mismatch assumes that the second sequence has at least as many members as the first sequence. If the two sequences are identical, mismatch returns a pair of iterators that point to the end of the first sequence and the corresponding location at which the comparison stopped in the second sequence.
The first version of mismatch checks members of a sequence for equality, while the second version lets you specify a comparison function. The comparison function must be a binary predicate.
The iterators i and j returned by mismatch are defined as follows:
j == first2 + (i - first1)
and i is the first iterator in the range [first1, last1) for which the appropriate one of the following conditions hold:
!(*i == *(first2 + (i - first1)))
or
binary_pred(*i, *(first2 + (i - first1))) == false
If all of the members in the two sequences match, mismatch returns a pair of last1 and first2 + (last1 - first1).
Complexity
At most last1 - first1 applications of the corresponding predicate are done.
Example
// // mismatch.cpp // #include <algorithm> #include <vector> #include <iostream.h> int main(void) { typedef vector<int>::iterator iterator; int d1[4] = {1,2,3,4}; int d2[4] = {1,3,2,4}; // Set up two vectors vector<int> vi1(d1,d1 + 4), vi2(d2,d2 + 4); // p1 will contain two iterators that point to the // first pair of elements that are different between // the two vectors pair<iterator, iterator> p1 = mismatch(vi1.begin(), vi1.end(), vi2.begin()); // find the first two elements such that an element in the // first vector is greater than the element in the second // vector. pair<iterator, iterator> p2 = mismatch(vi1.begin(), vi1.end(), vi2.begin(), less_equal<int>()); // Output results cout << *p1.first << ", " << *p1.second << endl; cout << *p2.first << ", " << *p2.second << endl; return 0; } Output : 2, 3 3, 2
Warning
If your compiler does not support default template parameters, then you need to always supply the Allocator template argument. For instance, you will need to write :
vector<int, allocator<int> >
instead of:
vector<int>
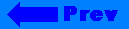
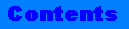
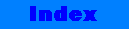
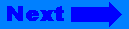
©Copyright 1996, Rogue Wave Software, Inc.