Personal tools
accumulate

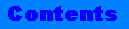
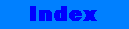
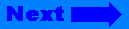
Click on the banner to return to the class reference home page.
accumulate
Generalized Numeric Operation
Summary
Accumulate all elements within a range into a single value.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <numeric> template <class InputIterator, class T> T accumulate (InputIterator first, InputIterator last, T init); template <class InputIterator, class T, class BinaryOperation> T accumulate (InputIterator first, InputIterator last, T init, BinaryOperation binary_op);
Description
accumulate applies a binary operation to init and each value in the range [first,last). The result of each operation is returned in init. This process aggregates the result of performing the operation on every element of the sequence into a single value.
Accumulation is done by initializing the accumulator acc with the initial value init and then modifying it with acc = acc + *i or acc = binary_op(acc, *i) for every iterator i in the range [first, last) in order. If the sequence is empty, accumulate returns init.
Complexity
accumulate performs exactly last-first applications of the binary operation (operator+ by default).
Example
// // accum.cpp // #include <numeric> //for accumulate #include <vector> //for vector #include <functional> //for times #include <iostream.h> int main() { // //Typedef for vector iterators // typedef vector<int>::iterator iterator; // //Initialize a vector using an array of ints // int d1[10] = {1,2,3,4,5,6,7,8,9,10}; vector<int> v1(d1, d1+10); // //Accumulate sums and products // int sum = accumulate(v1.begin(), v1.end(), 0); int prod = accumulate(v1.begin(), v1.end(), 1, times<int>()); // //Output the results // cout << "For the series: "; for(iterator i = v1.begin(); i != v1.end(); i++) cout << *i << " "; cout << " where N = 10." << endl; cout << "The sum = (N*N + N)/2 = " << sum << endl; cout << "The product = N! = " << prod << endl; return 0; } Output : For the series: 1 2 3 4 5 6 7 8 9 10 where N = 10. The sum = (N*N + N)/2 = 55 The product = N! = 3628800
Warnings
If your compiler does not support default template parameters then you need to always supply the Allocator template argument. For instance you'll have to write:
vector<int,allocator<int> >
instead of:
vector<int>
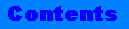
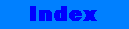
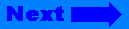
©Copyright 1996, Rogue Wave Software, Inc.