Personal tools
char_traits

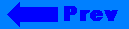
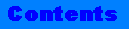
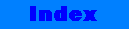
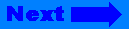
Click on the banner to return to the class reference home page.
char_traits
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Types Default-Values
- Value Functions
- Test Functions
- Conversion Functions
- See Also
- Standards Conformance
Summary
A traits class providing types and operations to the basic_string container and iostream classes.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | |
char_type int_type off_type pos_type |
state_type |
Member Functions | |
assign() compare() copy() eof() eq() eq_int_type() find() length() lt() move() |
not_eof() to_char_type() to_int_type() |
Synopsis
#include <string> template<class charT> struct char_traits
Description
The template structure char_traits<charT> defines the types and functions necessary to implement the iostreams and string template classes. It is templatized on charT, which represents the character container type. Each specialized version of char_traits<charT> provides the default definitions corresponding to the specialized character container type.
Users have to provide specialization for char_traits if they use other character types than char and wchar_t.
Interface
template<class charT> struct char_traits { typedef charT char_type; typedef INT_T int_type; typedef POS_T pos_type; typedef OFF_T off_type; typedef STATE_T state_type; static char_type to_char_type(const int_type&); static int_type to_int_type(const char_type&); static bool eq(const char_type&,const char_type& ); static bool eq_int_type(const int_type&,const int_type&); static int_type eof(); static int_type not_eof(const int_type&); static void assign(char_type&,const char_type&); static bool lt(const char_type&,const char_type&); static int compare(const char_type*,const char_type*,size_t); static size_t length(const char_type*); static const char_type* find(const char_type*,int n,const char_type&); static char_type* move(char_type*,const char_type*,size_t); static char_type* copy(char_type*,const char_type*, size_t); static char_type* assign(char_type*,size_t,const char_type&); };
Types
char_type
The type char_type represents the character container type. It must be convertible to int_type.
int_type
The type int_type is another character container type which can also hold an end-of-file value. It is used as the return type of some of the iostream class member functions. If char_type is either char or wchar_t, int_type is int or wint_t, respectively.
off_type
a signed displacement, measured in characters, from a specified position within a sequence.
an absolute position within a sequence.
The type off_type represents offsets to positional information. It is used to represent:
The value off_type(-1) can be used as an error indicator. Value of type off_type can be converted to type pos_type, but no validity of the resulting pos_type value is ensured.
If char_type is either char or wchar_t, off_type is streamoff or wstreamoff, respectively.
pos_type
The type pos_type describes an object that can store all the information necessary to restore an arbitrary sequence to a previous stream position and conversion state. The conversion pos_type(off_type(-1)) constructs the invalid pos_type value to signal error.
If char_type is either char or wchar_t, pos_type is streampos or wstreampos, respectively.
state_type
The type state_type holds the conversion state, and is compatible with the function locale::codecvt().
If char_type is either char or wchar_t, state_type is mbstate_t.
Types Default-Values
specialization type |
on char |
on wchar_t |
char_type |
char |
wchar_t |
int_type |
int |
wint_t |
off_type |
streamoff |
wstreamoff |
pos_type |
streampos |
wstreampos |
state_type |
mbstate_t |
mbstate_t |
Value Functions
void assign(char_type& c1, const char_type& c2);
Assigns one character value to another. The value of c2 is assigned to c1.
char_type* assign(char_type* s,size_t n,const char_type& a);
Assigns one character value to n elements of a character array. The value of a is assigned to n elements of s.
char_type* copy(char_type* s1, const char_type* s2, size_t n);
Copies n characters from the object pointed at by s1 into the object pointed at by s2. The ranges of (s1,s1+n) and (s2,s2+n) may not overlap.
int_type eof();
Returns an int_type value which represents the end-of-file. It is returned by several functions to indicate end-of-file state, or to indicate an invalid return value.
const char_type* find(const char_type* s, int n, const char_type& a);
Looks for the value of a in s. Only n elements of s are examined. Returns a pointer to the matched element if one is found. Otherwise returns a pointer to the n element in s.
size_t length(const char_type* s);
Returns the length of a null terminated character string pointed at by s.
char_type* move(char_type* s1, const char_type* s2, size_t n);
Moves n characters from the object pointed at by s1 into the object pointed at by s2. The ranges of (s1,s1+n) and (s2,s2+n) may overlap.
int_type not_eof(const int_type& c);
Returns a value which is not equal to the end-of-file value.
Test Functions
int compare(const char_type* s1,const char_type* s2,size_t n);
Compares n values from s1 with n values from s2. Returns 1 if s1 is greater than s2, -1 if s1 is less than s2, or 0 if they are equal.
bool eq(const char_type& c1, const char_type& c2);
Returns true if c1 and c2 represent the same character.
bool eq_int_type(const int_type& c1, const int_type& c2);
Returns true if c1 and c2 represents the same character.
bool lt(const char_type& c1,const char_type& c2);
Returns true if c1 is less than c2.
Conversion Functions
char_type to_char_type(const int_type& c);
Converts a valid character represented by a value of type int_type to the corresponding char_type value.
int_type to_int_type(const char_type& c);
Converts a valid character represented by a value of type char_type to the corresponding int_type value.
See Also
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Section 21.1.4, 21.1.5, 27.1.2.
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
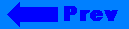
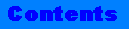
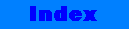
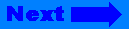
©Copyright 1996, Rogue Wave Software, Inc.