Personal tools
max

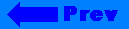
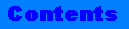
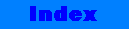
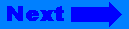
Click on the banner to return to the class reference home page.
max
Algorithm
Summary
Find and return the maximum of a pair of values
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class T> const T& max(const T&, const T&); template <class T, class Compare> const T& max(const T&, const T&, Compare);
Description
The max algorithm determines and returns the maximum of a pair of values. The optional argument Compare defines a comparison function that can be used in place of the default operator<. This function can be used with all the datatypes provided by the standard library.
max returns the first argument when the arguments are equal.
Example
// // max.cpp // #include <algorithm> #include <iostream.h> #include <iostream.h> int main(void) { double d1 = 10.0, d2 = 20.0; // Find minimum double val1 = min(d1, d2); // val1 = 10.0 // the greater comparator returns the greater of the // two values. double val2 = min(d1, d2, greater<double>()); // val2 = 20.0; // Find maximum double val3 = max(d1, d2); // val3 = 20.0; // the less comparator returns the smaller of the two values. // Note that, like every comparison in the STL, max is // defined in terms of the < operator, so using less here // is the same as using the max algorithm with a default // comparator. double val4 = max(d1, d2, less<double>()); // val4 = 20 cout << val1 << " " << val2 << " " << val3 << " " << val4 << endl; return 0; } Output : 10 20 20 20
See Also
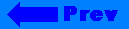
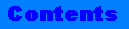
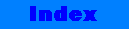
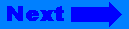
©Copyright 1996, Rogue Wave Software, Inc.